使用多线程/进程和System V信号量解决生产者消费者问题:有一个长度为N的缓冲池(共享内存)被生产者和消费者共同使用。只要缓冲池未满,生产者就可以将消息送入缓冲池;只要缓冲池不空,消费者便可从缓冲池中取走一个消息。生产者向缓冲池放入消息的同时,消费者不能操作缓冲池,反之亦然。(提示:两个C文件,consumer.c,producer.c),实验任务结果测试图文末给出。
时间: 2024-02-12 07:07:41 浏览: 46

System V共享内存与信号量综合应用之生产者与消费者问题解决

好的,这是一个经典的生产者消费者问题,可以使用多线程/进程和System V信号量来解决。
首先,我们需要定义缓冲池的结构体,其中包含一个指向消息的指针数组,以及两个指向当前可用消息和可用缓冲区的指针:
```c
#define N 10 // 缓冲池大小
struct buffer {
int in; // 当前可用缓冲区
int out; // 当前可用消息
char *msgs[N]; // 消息指针数组
};
```
接下来,我们需要定义两个函数,分别代表生产者和消费者的行为。这里我们只需要模拟他们向缓冲池中放入和取出消息即可:
```c
void producer(struct buffer *buf, char *msg) {
// 等待缓冲池未满
// P操作
// ...
// 放入消息
buf->msgs[buf->in] = msg;
buf->in = (buf->in + 1) % N;
// 通知缓冲池非空
// V操作
// ...
}
char *consumer(struct buffer *buf) {
char *msg;
// 等待缓冲池非空
// P操作
// ...
// 取出消息
msg = buf->msgs[buf->out];
buf->out = (buf->out + 1) % N;
// 通知缓冲池未满
// V操作
// ...
return msg;
}
```
最后,我们需要在main函数中创建生产者和消费者线程/进程,并使用System V信号量来保证它们的互斥访问:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <pthread.h>
#define KEY 1234
union semun {
int val;
struct semid_ds *buf;
unsigned short *array;
};
int semid;
void P(int semid, int index) {
struct sembuf sem;
sem.sem_num = index;
sem.sem_op = -1;
sem.sem_flg = 0;
semop(semid, &sem, 1);
}
void V(int semid, int index) {
struct sembuf sem;
sem.sem_num = index;
sem.sem_op = 1;
sem.sem_flg = 0;
semop(semid, &sem, 1);
}
void *producer_thread(void *arg) {
struct buffer *buf = (struct buffer *)arg;
// 生产消息
char *msg = "Hello, world!";
while (1) {
// 等待缓冲池未满
P(semid, 0);
// 放入消息
producer(buf, msg);
printf("Producer: %s\n", msg);
// 通知缓冲池非空
V(semid, 1);
}
return NULL;
}
void *consumer_thread(void *arg) {
struct buffer *buf = (struct buffer *)arg;
char *msg;
while (1) {
// 等待缓冲池非空
P(semid, 1);
// 取出消息
msg = consumer(buf);
printf("Consumer: %s\n", msg);
// 通知缓冲池未满
V(semid, 0);
}
return NULL;
}
int main() {
// 创建共享内存
int shmid = shmget(IPC_PRIVATE, sizeof(struct buffer), IPC_CREAT | 0666);
if (shmid < 0) {
perror("shmget");
exit(1);
}
// 连接共享内存
struct buffer *buf = shmat(shmid, NULL, 0);
if (buf == (void *)-1) {
perror("shmat");
exit(1);
}
// 初始化缓冲池
buf->in = 0;
buf->out = 0;
// 创建System V信号量
semid = semget(KEY, 2, IPC_CREAT | 0666);
if (semid < 0) {
perror("semget");
exit(1);
}
// 初始化信号量
union semun arg;
arg.val = N;
semctl(semid, 0, SETVAL, arg);
semctl(semid, 1, SETVAL, arg);
// 创建生产者和消费者线程
pthread_t producer_tid, consumer_tid;
pthread_create(&producer_tid, NULL, producer_thread, buf);
pthread_create(&consumer_tid, NULL, consumer_thread, buf);
// 等待线程结束
pthread_join(producer_tid, NULL);
pthread_join(consumer_tid, NULL);
// 删除信号量
semctl(semid, 0, IPC_RMID, arg);
// 断开共享内存连接
shmdt(buf);
// 删除共享内存
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
以上就是使用多线程/进程和System V信号量解决生产者消费者问题的完整代码。注意,这里使用了pthread库创建线程,如果使用进程则需要使用fork函数创建进程。同时,也需要注意System V信号量的使用,P操作和V操作分别对应着信号量值的减少和增加。
阅读全文
相关推荐
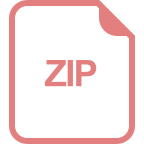


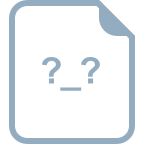
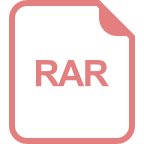
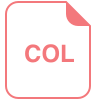
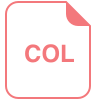

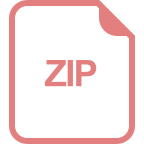
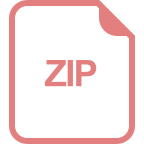
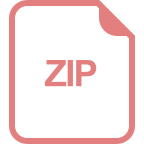
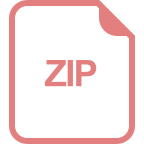
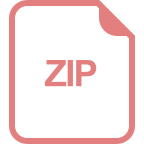
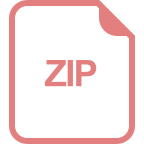
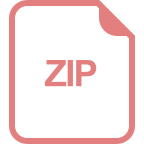
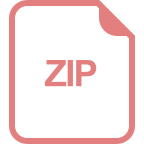
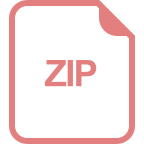