C语言写一个函数能够输入IP地址,端口,发包大小,发送时长以进行通信
时间: 2024-05-12 13:18:07 浏览: 94
你可以使用以下 C 语言代码来实现输入 IP 地址、端口、发包大小、发送时长以进行通信的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <time.h>
void send_packets(char *ip_addr, int port, int packet_size, int send_duration) {
// 创建 socket
int sockfd = socket(AF_INET, SOCK_DGRAM, 0);
if (sockfd < 0) {
printf("Error creating socket\n");
return;
}
// 设置目标地址
struct sockaddr_in dest_addr;
memset(&dest_addr, 0, sizeof(dest_addr));
dest_addr.sin_family = AF_INET;
dest_addr.sin_port = htons(port);
if (inet_pton(AF_INET, ip_addr, &dest_addr.sin_addr) <= 0) {
printf("Invalid IP address\n");
close(sockfd);
return;
}
// 生成随机数据包
char packet[packet_size];
for (int i = 0; i < packet_size; i++) {
packet[i] = rand() % 256;
}
// 发送数据包
time_t start_time = time(NULL);
while (time(NULL) < start_time + send_duration) {
if (sendto(sockfd, packet, packet_size, 0, (struct sockaddr *)&dest_addr, sizeof(dest_addr)) < 0) {
printf("Error sending packet\n");
break;
}
}
// 关闭 socket
close(sockfd);
}
int main() {
// 输入 IP 地址、端口、发包大小、发送时长
char ip_addr[16] = {0};
int port, packet_size, send_duration;
printf("Enter IP address: ");
scanf("%s", ip_addr);
printf("Enter port: ");
scanf("%d", &port);
printf("Enter packet size: ");
scanf("%d", &packet_size);
printf("Enter send duration (in seconds): ");
scanf("%d", &send_duration);
// 发送数据包
send_packets(ip_addr, port, packet_size, send_duration);
return 0;
}
```
希望对您有帮助!
阅读全文
相关推荐
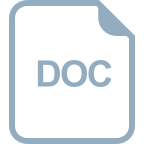
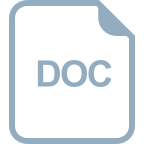
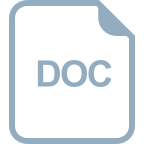

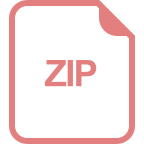
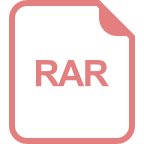
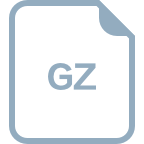
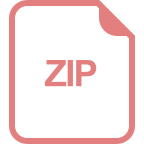
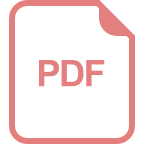





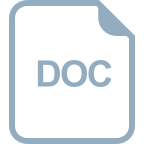
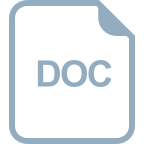
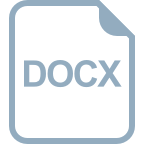