在 OC中,如何 NSString 转成 const char *
时间: 2024-03-09 13:46:22 浏览: 18
在 Objective-C 中,可以通过以下代码将 NSString 转换为 const char *:
```objective-c
NSString *str = @"hello world";
const char *cString = [str cStringUsingEncoding:NSUTF8StringEncoding];
```
这里使用了 NSString 的 `cStringUsingEncoding:` 方法将字符串转换为 C 语言风格的字符串。使用 `NSUTF8StringEncoding` 参数来指定字符编码,如果需要使用其他编码,可以在此处进行更改。
相关问题
在 Swift 中,如何 NSString 转成 const char *
在 Swift 中,可以通过以下代码将 NSString 转换为 const char *:
```swift
let str: NSString = "hello world"
let cString = str.cString(using: String.Encoding.utf8)
```
这里使用了 NSString 的 `cString(using:)` 方法将字符串转换为 C 语言风格的字符串。使用 `String.Encoding.utf8` 参数来指定字符编码,如果需要使用其他编码,可以在此处进行更改。
在oc中,如何将const char *转换成NSString
在 Objective-C 中,可以使用 `NSString` 的 `stringWithUTF8String:` 方法将 `const char *` 类型的字符串转换为 `NSString` 对象,示例如下:
```objective-c
const char *cString = "hello world";
NSString *str = [NSString stringWithUTF8String:cString];
```
在上面的示例中,我们将 `const char *` 类型的字符串 `"hello world"` 转换为 `NSString` 对象 `str`。
需要注意的是,如果 `const char *` 类型的字符串是使用动态内存分配函数(如 `malloc`、`calloc`、`realloc` 等)分配的,则需要手动释放它们的内存。例如:
```objective-c
char *cString = malloc(sizeof(char) * 12);
strcpy(cString, "hello world");
NSString *str = [NSString stringWithUTF8String:cString];
free(cString);
```
在上面的示例中,我们使用 `malloc` 分配了一块内存来存储字符串 `"hello world"`,然后使用 `strcpy` 将字符串复制到该内存中。最后,我们将 `cString` 字符串转换为 `NSString` 对象 `str`,并手动释放了 `cString` 字符串所占用的内存。
相关推荐
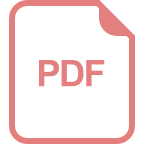
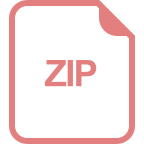
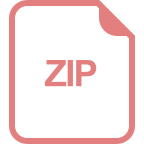













