pyqt QMessageBox
时间: 2023-10-15 15:04:25 浏览: 28
QMessageBox is a pre-defined dialog box in PyQt5 that is used to display messages, warnings, errors, information, etc. to the user. It is a pre-built class that provides a standard interface for displaying messages to the user.
Here is an example of using QMessageBox to display a message box with some text and an OK button:
```
from PyQt5.QtWidgets import QMessageBox, QApplication
app = QApplication([])
msgBox = QMessageBox()
msgBox.setText("Hello, this is a message box!")
msgBox.exec_()
```
In this example, we create a QMessageBox object and set its text to "Hello, this is a message box!". We then call the exec_() method to display the message box to the user.
QMessageBox has several other methods that can be used to customize the appearance and behavior of the message box. For example, you can set the icon, add buttons, or set a default button. Here is an example that demonstrates some of these options:
```
from PyQt5.QtWidgets import QMessageBox, QApplication
app = QApplication([])
msgBox = QMessageBox()
msgBox.setIcon(QMessageBox.Information)
msgBox.setText("Are you sure you want to exit?")
msgBox.setInformativeText("All unsaved changes will be lost.")
msgBox.addButton(QMessageBox.Save)
msgBox.addButton(QMessageBox.Discard)
msgBox.addButton(QMessageBox.Cancel)
msgBox.setDefaultButton(QMessageBox.Save)
response = msgBox.exec_()
if response == QMessageBox.Save:
saveChanges()
elif response == QMessageBox.Discard:
discardChanges()
else:
cancel()
```
In this example, we create a message box with an information icon and a text message. We also add three buttons (Save, Discard, and Cancel) and set the default button to Save. We then call the exec_() method to display the message box and wait for the user's response.
Depending on the button the user clicks, we can call different functions to handle the response. For example, if the user clicks the Save button, we can call a function to save the changes. If the user clicks the Cancel button, we can simply close the message box without doing anything.
相关推荐
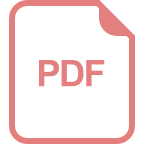
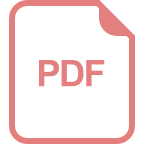
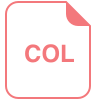













