protected float doEstimatePreference(long theUserID, long[] theNeighborhood, long itemID) throws Exception { if (theNeighborhood.length == 0) { return Float.NaN; } DataModel dataModel = getDataModel(); double preference = 0.0; double totalSimilarity = 0.0; int count = 0; for (long userID : theNeighborhood) { if (userID != theUserID) { Float pref = dataModel.getPreferenceValue(userID, itemID); if (pref != null) { double theSimilarity = similarity.userSimilarity(theUserID, userID); if (!Double.isNaN(theSimilarity)) { preference += theSimilarity * pref; totalSimilarity += theSimilarity; count++; } } } } if (count <= 0) { return Float.NaN; } return (float) (preference / totalSimilarity); } 解释代码
时间: 2023-12-06 18:40:35 浏览: 52
这段代码是协同过滤推荐算法中的基于用户的邻域方法,用于预测用户对某个物品的评分。具体来说,该方法接收三个参数:用户ID、邻域用户ID数组和物品ID,返回一个float类型的预测评分值。
首先,如果邻域用户ID数组为空,则返回NaN表示无法预测。接着,获取数据模型对象并初始化预测评分值preference、相似度总和totalSimilarity和邻域用户数量count为0。
然后,遍历邻域用户ID数组,对于每个邻域用户ID,排除自身,通过数据模型获取该用户对物品的评分值pref。如果评分值不为空,则计算用户相似度theSimilarity,如果相似度不是NaN,则将相似度乘以评分值加到预测评分值preference,将相似度累加到相似度总和totalSimilarity,邻域用户数量加1。
最后,如果邻域用户数量小于等于0,则返回NaN表示无法预测。否则,将预测评分值preference除以相似度总和totalSimilarity作为最终的预测评分值返回。
相关问题
private final class Estimator implements TopItems.Estimator<Long> { private final long theUserID; private final long[] theNeighborhood; Estimator(long theUserID, long[] theNeighborhood) { this.theUserID = theUserID; this.theNeighborhood = theNeighborhood; } @Override public double estimate(Long itemID) throws Exception { return GenericUserBasedRecommender.this.doEstimatePreference(theUserID, theNeighborhood, itemID); } } 解释代码
这段代码是一个私有内部类 `Estimator`,实现了 `TopItems.Estimator` 接口,泛型参数为 `Long`。该接口用于计算某个用户对某个物品的兴趣度或评分。
在 `Estimator` 类中,有两个成员变量 `theUserID` 和 `theNeighborhood` 分别表示目标用户ID和与目标用户相似的一组用户ID。
在 `estimate` 方法中,调用了 `GenericUserBasedRecommender` 类中的 `doEstimatePreference` 方法,计算目标用户对某个物品的预测评分,并返回该评分。这个方法的实现会根据用户相似度和用户历史评分等因素来计算预测评分。
protected FastIDSet getAllOtherItems(long[] theNeighborhood, long theUserID, boolean includeKnownItems) throws Exception { DataModel dataModel = getDataModel(); FastIDSet possibleItemIDs = new FastIDSet(); for (long userID : theNeighborhood) { possibleItemIDs.addAll(dataModel.getItemIDsFromUser(userID)); } if (!includeKnownItems) { possibleItemIDs.removeAll(dataModel.getItemIDsFromUser(theUserID)); } return possibleItemIDs; } private final class Estimator implements TopItems.Estimator<Long> { private final long theUserID; private final long[] theNeighborhood; Estimator(long theUserID, long[] theNeighborhood) { this.theUserID = theUserID; this.theNeighborhood = theNeighborhood; } @Override public double estimate(Long itemID) throws Exception { return GenericUserBasedRecommender.this.doEstimatePreference(theUserID, theNeighborhood, itemID); } } 解释代码
这段代码是一个基于用户的推荐算法中的一个方法,其中包含了一个内部类 Estimator。该方法的作用是获取用户邻居中所有的物品,并返回一个 FastIDSet 类型的对象。其中的 theNeighborhood 参数是一个 long 类型数组,表示用户邻居的 ID;theUserID 参数表示当前用户的 ID;includeKnownItems 参数表示是否包含当前用户已经评分过的物品。
Estimator 类是一个实现了 TopItems.Estimator 接口的内部类,它的作用是计算一个物品的预测得分。该类包含了两个成员变量 theUserID 和 theNeighborhood,分别表示当前用户的 ID 和用户邻居的 ID 数组。该类实现了 TopItems.Estimator 接口中的 estimate 方法,该方法接受一个 Long 类型的 itemID 参数,表示要计算预测得分的物品的 ID。该方法调用了 GenericUserBasedRecommender 类的 doEstimatePreference 方法来计算物品的预测得分,并返回该得分。
阅读全文
相关推荐
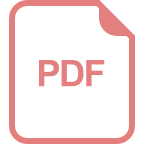
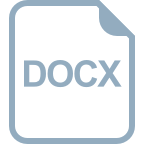
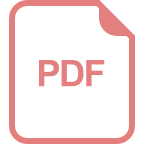
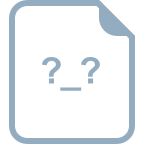
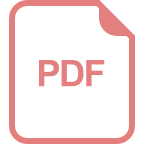
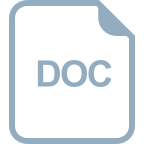
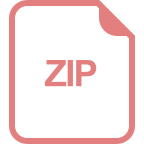
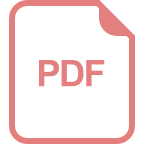
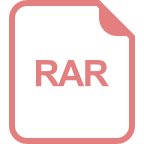
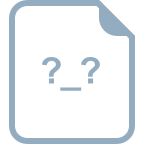
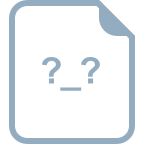
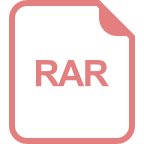
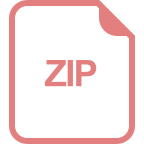
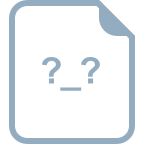
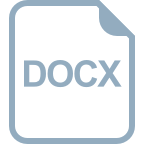
