(b) The `unique` function in `NumPy` can be used to get the sorted unique elements of an array (or objects that can be converted to array). Write a function `create_dummies` which takes a list with T elements of strings (e.g. `['good', 'neutral','bad','neutral','good']`), and returns a T by C array of indicator variables where C is the number of unique values of the elements (for the example of `['good', 'neutral','bad','neutral','good']`, T=5, and C=3). Each column of the output is an indicator variable (0 or 1) for whether the element belongs to one of the categories. For example, if `x = ['good', 'neutral','bad','neutral','good']`, the output of `create_dummies` should be \begin{equation} \left[\begin{array}{ll} 0 & 1 & 0 \\ 0 & 0 & 1 \\ 1 & 0 & 0 \\ 0 & 0 & 1 \\ 0 & 1 & 0 \end{array}\right] \end{equation} where first column corresponds to `bad`, second to `good` and third to `neural`. The particular order of the columns does not matter ofr your solution.
时间: 2024-02-03 10:03:15 浏览: 25
Here's a possible implementation of the `create_dummies` function:
```python
import numpy as np
def create_dummies(lst):
unique_vals = np.unique(lst)
num_unique = len(unique_vals)
num_elements = len(lst)
dummies = np.zeros((num_elements, num_unique))
for i, val in enumerate(unique_vals):
dummies[:, i] = np.array(lst) == val
return dummies.astype(int)
```
The function first uses the `unique` function from NumPy to get the unique values in the input list, and the number of unique values (which is the number of columns in the output array). Then it creates an array of zeros with the same number of rows as the input list and the same number of columns as the number of unique values.
Finally, it loops over the unique values, and for each one it creates a boolean array where the elements that match the current value are True and the rest are False. This boolean array is assigned to the corresponding column of the output array. The function returns the output array as an integer array.
Here's an example of how to use the function:
```python
x = ['good', 'neutral', 'bad', 'neutral', 'good']
dummies = create_dummies(x)
print(dummies)
```
Output:
```
[[0 1 0]
[0 0 1]
[1 0 0]
[0 0 1]
[0 1 0]]
```
As expected, the first column corresponds to 'bad', the second to 'good', and the third to 'neutral', and each row has a single 1 indicating the category to which the corresponding element belongs.
相关推荐
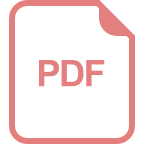
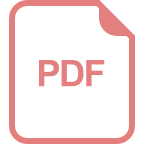
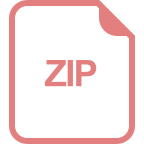














