用c++ 实现车牌号代码
时间: 2024-03-07 14:46:25 浏览: 155
以下是一个简单的 C++ 代码示例,用于实现车牌号的输入和输出:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string licensePlate;
cout << "请输入车牌号:" << endl;
cin >> licensePlate;
cout << "您输入的车牌号是:" << licensePlate << endl;
return 0;
}
```
这个程序提醒用户输入车牌号,然后将该车牌号存储在一个字符串变量 `licensePlate` 中,最后输出该车牌号。
相关问题
用c++ 实现随机车牌号代码
以下是一个简单的 C++ 代码示例,用于生成随机车牌号:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
srand(time(0)); // 初始化随机数生成器
// 随机生成省份简称
string province[] = {"京", "津", "沪", "渝", "冀", "豫", "云", "辽", "黑", "湘", "皖", "鲁", "新", "苏", "浙", "赣", "鄂", "桂", "甘", "晋", "蒙", "陕", "吉", "闽", "贵", "粤", "青", "藏", "川", "宁", "琼"};
string provinceCode = province[rand() % 30];
// 随机生成字母
string letter[] = {"A", "B", "C", "D", "E", "F", "G", "H", "J", "K", "L", "M", "N", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"};
string letterCode = letter[rand() % 24];
// 随机生成数字
string number[] = {"0", "1", "2", "3", "4", "5", "6", "7", "8", "9"};
string numberCode = number[rand() % 10];
// 生成车牌号
string licensePlate = provinceCode + letterCode + numberCode + number[rand() % 10] + number[rand() % 10] + number[rand() % 10] + number[rand() % 10];
cout << "随机生成的车牌号为:" << licensePlate << endl;
return 0;
}
```
这个程序使用 `srand(time(0))` 初始化随机数生成器,并使用三个数组来随机生成车牌号的省份简称、字母和数字。程序生成的车牌号的格式为“省份简称 + 字母 + 数字 + 4位数字”,例如“京A1234”。
用c++ 实现输入名字随机车牌号代码
好的,以下是一个简单的 C++ 代码实现,可以生成随机车牌号:
```cpp
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
string generateLicensePlate(string name) {
string plate = "";
srand(time(0));
for (int i = 0; i < 6; i++) {
if (i < 2) {
plate += toupper(name[i]);
} else {
plate += to_string(rand() % 10);
}
}
return plate;
}
int main() {
string name;
cout << "请输入名字:";
cin >> name;
string licensePlate = generateLicensePlate(name);
cout << "您的车牌号为:" << licensePlate << endl;
return 0;
}
```
这个程序首先让用户输入名字,然后调用 `generateLicensePlate` 函数生成随机车牌号。这个函数采用了当前时间作为随机数种子,然后生成一个 6 位的车牌号,前两位是名字的前两个字母(大写),后四位是 0 到 9 的随机数字组成。最后,程序将生成的车牌号输出给用户。
阅读全文
相关推荐
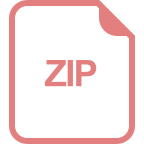
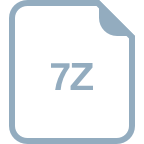
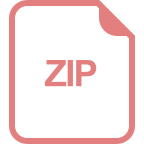













