vue+elementUI+vuex待办事项 包含以下功能:一、新增待办事项 二、编辑待办事项 三、一键清空所有已办事项 四、一键标记所有待办为已办 五、一键标记所有已办为待办 六、计算待办事项数 七、计算已办事项数
时间: 2023-11-08 10:41:25 浏览: 58
八、按照时间顺序排序待办事项 九、按照时间顺序排序已办事项 十、搜索待办事项 十一、筛选待办事项状态(已办/待办) 十二、支持多种主题色切换 十三、支持多语言切换(中文/英文等) 十四、支持导出待办事项列表为Excel或PDF格式 十五、支持分享待办事项给其他人 十六、支持提醒功能,可以设置提醒时间和方式(邮件/短信/手机推送等) 十七、支持多用户登录和权限管理,管理员可以添加/删除用户和分配权限。
相关问题
使用vue+elementUI+vuex 代码实现一个待办事项页面并包含以下功能 1.新增待办事项
2.删除待办事项 3.标记已完成待办事项 4.编辑待办事项
首先,需要安装Vue、ElementUI和Vuex。
```
npm install vue
npm install element-ui
npm install vuex
```
在main.js中引入Vue、ElementUI和Vuex:
```javascript
import Vue from 'vue'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
import Vuex from 'vuex'
import App from './App.vue'
Vue.use(ElementUI)
Vue.use(Vuex)
Vue.config.productionTip = false
new Vue({
render: h => h(App),
}).$mount('#app')
```
接下来,创建store.js文件,定义state、mutations、actions和getters:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
todos: [
{ id: 1, text: '写代码', done: false },
{ id: 2, text: '看电影', done: true },
{ id: 3, text: '做饭', done: false }
]
},
mutations: {
addTodo (state, todo) {
state.todos.push(todo)
},
deleteTodo (state, id) {
state.todos = state.todos.filter(todo => todo.id !== id)
},
toggleTodo (state, id) {
state.todos = state.todos.map(todo => {
if (todo.id === id) {
todo.done = !todo.done
}
return todo
})
},
editTodo (state, todo) {
state.todos = state.todos.map(item => (item.id === todo.id ? todo : item))
}
},
actions: {
addTodo ({ commit }, todo) {
commit('addTodo', todo)
},
deleteTodo ({ commit }, id) {
commit('deleteTodo', id)
},
toggleTodo ({ commit }, id) {
commit('toggleTodo', id)
},
editTodo ({ commit }, todo) {
commit('editTodo', todo)
}
},
getters: {
todos: state => state.todos,
doneTodos: state => state.todos.filter(todo => todo.done),
activeTodos: state => state.todos.filter(todo => !todo.done)
}
})
```
在App.vue中,使用ElementUI的组件实现待办事项页面:
```vue
<template>
<div class="container">
<h1 class="title">Todo List</h1>
<div class="add-todo">
<el-input v-model="newTodo" placeholder="请输入待办事项"></el-input>
<el-button type="primary" @click="addTodo">添加</el-button>
</div>
<el-divider></el-divider>
<el-card v-for="todo in activeTodos" :key="todo.id" class="todo-card">
<div class="todo-content">
<el-checkbox v-model="todo.done" @change="toggleTodo" class="todo-checkbox">{{ todo.text }}</el-checkbox>
<el-button-group>
<el-button type="text" @click="editTodo">编辑</el-button>
<el-button type="text" @click="deleteTodo">删除</el-button>
</el-button-group>
</div>
</el-card>
<el-divider></el-divider>
<h2 class="subtitle">已完成</h2>
<el-card v-for="todo in doneTodos" :key="todo.id" class="todo-card">
<div class="todo-content">
<el-checkbox v-model="todo.done" @change="toggleTodo" class="todo-checkbox">{{ todo.text }}</el-checkbox>
<el-button-group>
<el-button type="text" @click="editTodo">编辑</el-button>
<el-button type="text" @click="deleteTodo">删除</el-button>
</el-button-group>
</div>
</el-card>
<el-dialog :visible.sync="editVisible" title="编辑待办事项">
<el-input v-model="editTodoText" placeholder="请输入待办事项"></el-input>
<div slot="footer">
<el-button @click="editVisible = false">取消</el-button>
<el-button type="primary" @click="saveEdit">保存</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
import { mapGetters, mapActions } from 'vuex'
export default {
name: 'App',
computed: {
...mapGetters(['activeTodos', 'doneTodos'])
},
data () {
return {
newTodo: '',
editTodoId: null,
editTodoText: '',
editVisible: false
}
},
methods: {
...mapActions(['addTodo', 'deleteTodo', 'toggleTodo', 'editTodo']),
addTodo () {
if (this.newTodo.trim()) {
this.addTodo({
id: Date.now(),
text: this.newTodo,
done: false
})
this.newTodo = ''
}
},
deleteTodo (todo) {
this.deleteTodo(todo.id)
},
toggleTodo (todo) {
this.toggleTodo(todo.id)
},
editTodo (todo) {
this.editTodoId = todo.id
this.editTodoText = todo.text
this.editVisible = true
},
saveEdit () {
if (this.editTodoText.trim()) {
this.editTodo({
id: this.editTodoId,
text: this.editTodoText,
done: false
})
this.editVisible = false
}
}
}
}
</script>
<style>
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
.title {
text-align: center;
margin-bottom: 20px;
}
.add-todo {
display: flex;
align-items: center;
margin-bottom: 20px;
}
.todo-card {
margin-bottom: 10px;
}
.todo-content {
display: flex;
align-items: center;
justify-content: space-between;
}
.todo-checkbox {
flex: 1;
margin-right: 10px;
}
.subtitle {
margin-top: 20px;
margin-bottom: 10px;
}
</style>
```
最后,在index.html中添加id为app的div:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Todo List</title>
</head>
<body>
<div id="app"></div>
<script src="./dist/js/app.js"></script>
</body>
</html>
```
这样,就完成了一个基于Vue、ElementUI和Vuex的待办事项页面。
vue+elementUI+vuex 实现一个手写签名的功能
好的,针对您的问题,您可以按照下面的步骤实现一个手写签名的功能:
1. 首先,在vue中引入elementUI和vuex
2. 创建一个签名组件,在组件中添加canvas标签来实现手写签名的功能
3. 在组件中添加一些方法来获取canvas的绘图上下文和设置样式等
4. 在vuex中添加一个state来存储签名的数据
5. 在组件中添加一些方法来对canvas进行绘制,并将绘制的数据保存到vuex中的state中
6. 在Vuex中添加一个action来将签名数据提交到后端进行保存
7. 在组件中添加一个按钮或者其他交互方式来触发提交签名数据的操作
以上7个步骤就可以实现一个简单的手写签名功能。当然,实现的方式还可以有很多种,但整体的思路是差不多的。希望以上回答能够帮助到您!
阅读全文
相关推荐
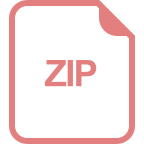
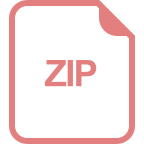
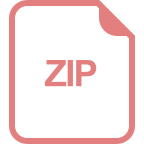
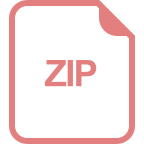
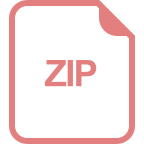
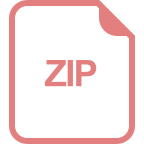
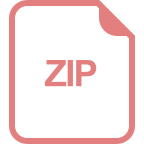
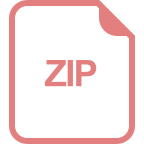
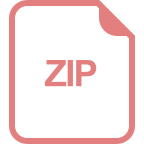
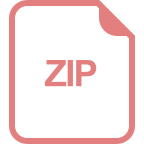
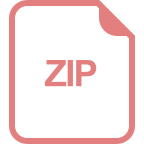
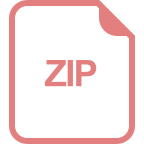

