把ov2640双目摄像头拍摄的RGB565格式照片上传至电脑的数据怎么用opencv转换为图片。给出在opencv上实现的代码
时间: 2023-06-06 11:08:29 浏览: 65
下面是将RGB565格式图片转换为可显示图像的OpenCV代码:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main()
{
// 读取RGB565格式的图片
string filename = "rgb565_image.bin";
int width = 640, height = 480;
ifstream file(filename, ios::in | ios::binary);
if (!file.is_open()) {
cerr << "Error opening file: " << filename << endl;
return -1;
}
vector<uchar> data(width * height * 2);
file.read(reinterpret_cast<char*>(data.data()), data.size());
file.close();
// 将RGB565数据转换为8位无符号整形
Mat img(height, width, CV_8UC3);
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j+=2) {
int index = (i * width + j) * 2;
uint16_t pixel = (static_cast<uint16_t>(data[index + 1]) << 8) | data[index];
img.at<Vec3b>(i, j)[0] = (pixel & 0x1F) << 3;
img.at<Vec3b>(i, j)[1] = ((pixel >> 5) & 0x3F) << 2;
img.at<Vec3b>(i, j)[2] = ((pixel >> 11) & 0x1F) << 3;
pixel = (static_cast<uint16_t>(data[index + 3]) << 8) | data[index + 2];
img.at<Vec3b>(i, j+1)[0] = (pixel & 0x1F) << 3;
img.at<Vec3b>(i, j+1)[1] = ((pixel >> 5) & 0x3F) << 2;
img.at<Vec3b>(i, j+1)[2] = ((pixel >> 11) & 0x1F) << 3;
}
}
// 显示图像
namedWindow("Image");
imshow("Image", img);
waitKey(0);
return 0;
}
```
其中,“rgb565_image.bin”为RGB565格式的图片文件名,宽度为640像素,高度为480像素。代码的主要部分是将RGB565数据转换为8位无符号整形,并创建OpenCV图像对象。最后,代码窗口中显示图像。
相关推荐
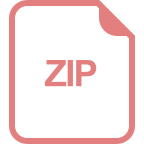
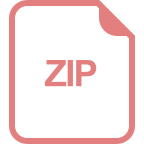
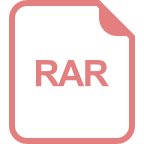














