Java实现直方图的均衡化与规范化完整代码
时间: 2023-09-01 18:10:46 浏览: 248
以下是Java实现直方图的均衡化与规范化的完整代码:
```java
import java.awt.Color;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class HistogramEqualization {
public static void main(String[] args) {
// 读取图像
BufferedImage image = null;
try {
File file = new File("input.jpg");
image = ImageIO.read(file);
} catch (Exception e) {
System.out.println("读取图像失败:" + e.getMessage());
return;
}
// 直方图均衡化
BufferedImage equalizedImage = equalizeHistogram(image);
// 直方图规范化
BufferedImage normalizedImage = normalizeHistogram(image, 100, 200);
// 保存图像
try {
File equalizedFile = new File("output_equalized.jpg");
ImageIO.write(equalizedImage, "jpg", equalizedFile);
File normalizedFile = new File("output_normalized.jpg");
ImageIO.write(normalizedImage, "jpg", normalizedFile);
} catch (Exception e) {
System.out.println("保存图像失败:" + e.getMessage());
return;
}
System.out.println("处理完成!");
}
// 直方图均衡化
public static BufferedImage equalizeHistogram(BufferedImage image) {
// 获取图像宽度和高度
int width = image.getWidth();
int height = image.getHeight();
// 计算直方图
int[] histogram = new int[256];
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color color = new Color(image.getRGB(i, j));
int gray = (color.getRed() + color.getGreen() + color.getBlue()) / 3;
histogram[gray]++;
}
}
// 计算灰度累计概率
double[] cumulativeProbability = new double[256];
int totalPixels = width * height;
for (int i = 0; i < 256; i++) {
cumulativeProbability[i] = (double) histogram[i] / totalPixels;
if (i > 0) {
cumulativeProbability[i] += cumulativeProbability[i - 1];
}
}
// 均衡化处理
BufferedImage equalizedImage = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color color = new Color(image.getRGB(i, j));
int gray = (color.getRed() + color.getGreen() + color.getBlue()) / 3;
int newGray = (int) (255 * cumulativeProbability[gray]);
Color newColor = new Color(newGray, newGray, newGray);
equalizedImage.setRGB(i, j, newColor.getRGB());
}
}
return equalizedImage;
}
// 直方图规范化
public static BufferedImage normalizeHistogram(BufferedImage image, int minGray, int maxGray) {
// 获取图像宽度和高度
int width = image.getWidth();
int height = image.getHeight();
// 计算直方图
int[] histogram = new int[256];
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color color = new Color(image.getRGB(i, j));
int gray = (color.getRed() + color.getGreen() + color.getBlue()) / 3;
histogram[gray]++;
}
}
// 计算最小灰度和最大灰度
int minGrayLevel = -1;
int maxGrayLevel = -1;
for (int i = 0; i < 256; i++) {
if (histogram[i] > 0) {
if (minGrayLevel == -1) {
minGrayLevel = i;
}
maxGrayLevel = i;
}
}
// 规范化处理
BufferedImage normalizedImage = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Color color = new Color(image.getRGB(i, j));
int gray = (color.getRed() + color.getGreen() + color.getBlue()) / 3;
int newGray = (int) (255 * (gray - minGray) / (double) (maxGray - minGray));
if (newGray < minGray) {
newGray = minGray;
}
if (newGray > maxGray) {
newGray = maxGray;
}
if (newGray < minGrayLevel) {
newGray = minGrayLevel;
}
if (newGray > maxGrayLevel) {
newGray = maxGrayLevel;
}
Color newColor = new Color(newGray, newGray, newGray);
normalizedImage.setRGB(i, j, newColor.getRGB());
}
}
return normalizedImage;
}
}
```
代码中包含了两个方法:
- `equalizeHistogram`:实现直方图均衡化。
输入参数为原始图像 `image`,返回值为均衡化后的图像 `equalizedImage`。
- `normalizeHistogram`:实现直方图规范化。
输入参数为原始图像 `image`、规范化后的最小灰度值 `minGray` 和最大灰度值 `maxGray`,返回值为规范化后的图像 `normalizedImage`。
阅读全文
相关推荐

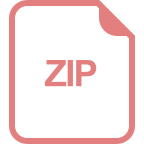
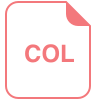
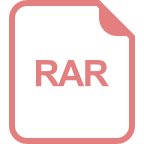
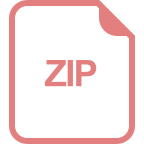
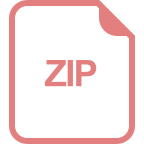
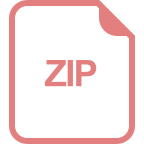
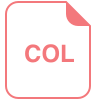
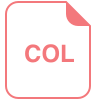
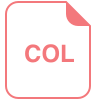
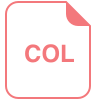
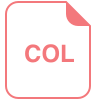
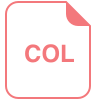
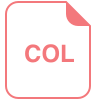
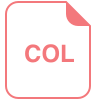
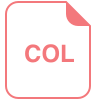
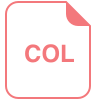