res = yolo_model(img)的res格式
时间: 2024-06-02 07:11:11 浏览: 128
在YOLO模型中,`res`通常是一个元组,包含三个元素:
1. `res[0]`是一个形状为`(batch_size, grid_size, grid_size, num_anchors, num_classes)`的张量,表示每个锚框对应每个类别的预测概率;
2. `res[1]`是一个形状为`(batch_size, grid_size, grid_size, num_anchors, 4)`的张量,表示每个锚框的位置和尺寸信息,四个元素分别为`(x,y,w,h)`;
3. `res[2]`是一个形状为`(batch_size, image_height, image_width, 3)`的张量,表示模型的预测结果图像,其中3个通道分别为`(r,g,b)`。
需要注意的是,`res`的具体格式可能会因不同YOLO模型的实现而有所不同。
相关问题
怎么用openvino部署yolo
以下是使用OpenVINO部署YOLO的步骤:
1. 下载YOLO的权重文件和配置文件,以及OpenVINO的模型优化工具。
2. 使用OpenVINO模型优化工具对YOLO的权重文件和配置文件进行转换,生成IR模型和XML文件。例如,使用以下命令:
```
mo.py --input_model yolov3.weights --config yolov3.cfg --output_dir yolo_v3 --data_type FP16
```
3. 将生成的IR模型和XML文件加载到OpenVINO中:
```python
from openvino.inference_engine import IENetwork, IEPlugin
model_xml = "yolo_v3.xml"
model_bin = "yolo_v3.bin"
plugin = IEPlugin(device="CPU")
net = IENetwork(model=model_xml, weights=model_bin)
exec_net = plugin.load(network=net)
```
4. 将输入图像转换为OpenVINO可接受的格式,并进行推理:
```python
import cv2
import numpy as np
# Load input image
img = cv2.imread("input.jpg")
# Convert image to OpenVINO format
input_blob = next(iter(net.inputs))
n, c, h, w = net.inputs[input_blob].shape
img = cv2.resize(img, (w, h))
img = img.transpose((2, 0, 1))
img = img.reshape((n, c, h, w))
# Run inference
res = exec_net.infer(inputs={input_blob: img})
```
5. 解析推理结果并绘制检测框:
```python
# Parse detection results
output_blob = next(iter(net.outputs))
outputs = res[output_blob]
boxes = []
for i in range(outputs.shape[2]):
conf = outputs[0, 0, i, 2]
if conf > 0.5:
x1 = int(outputs[0, 0, i, 3] * w)
y1 = int(outputs[0, 0, i, 4] * h)
x2 = int(outputs[0, 0, i, 5] * w)
y2 = int(outputs[0, 0, i, 6] * h)
boxes.append((x1, y1, x2, y2))
# Draw detection boxes
for box in boxes:
cv2.rectangle(img, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), 2)
# Show result
cv2.imshow("result", img)
cv2.waitKey(0)
```
这样就可以使用OpenVINO对YOLO进行推理了。
阅读全文
相关推荐
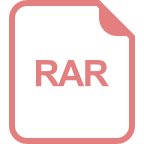
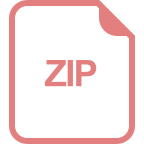
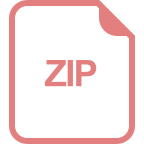
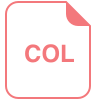
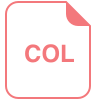
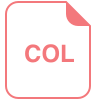
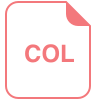
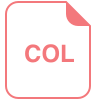
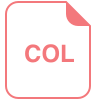
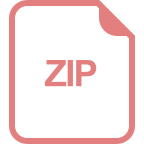
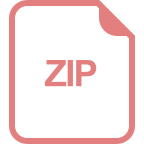