pyqt5 qtoolbar按钮间距
时间: 2023-06-21 11:13:27 浏览: 119
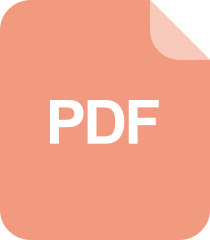
Python中PyQt5/PySide2的按钮控件使用实例
可以使用QToolBar的setContentsMargins方法来设置工具栏中按钮之间的间距。示例代码如下:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QToolBar, QAction
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
toolbar = QToolBar()
self.addToolBar(toolbar)
toolbar.setContentsMargins(10, 10, 10, 10) # 设置按钮间距
action1 = QAction('Action 1', self)
action2 = QAction('Action 2', self)
toolbar.addAction(action1)
toolbar.addAction(action2)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在上面的代码中,我们使用了setContentsMargins方法来设置工具栏中按钮之间的间距,代码中设置了一个10像素的间距。
阅读全文
相关推荐
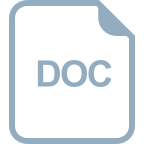
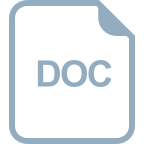



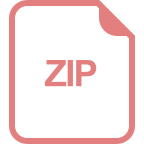
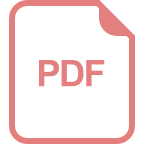
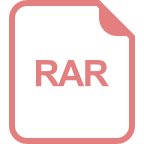
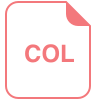
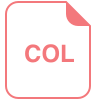
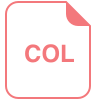


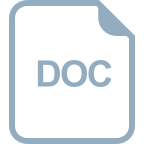
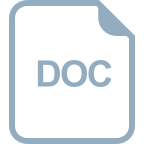
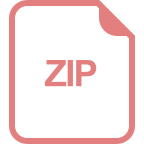