用warpper类写一个小程序
时间: 2023-06-09 14:02:32 浏览: 81
好的,我明白了。以下是一个用wrapper类编写的Python小程序示例:
```python
class Wrapper:
def __init__(self, func):
self.func = func
def __call__(self, *args, **kwargs):
print("Function is being executed...")
return self.func(*args, **kwargs)
@Wrapper
def add(a, b):
return a + b
result = add(1, 2)
print(result)
```
这个程序定义了一个Wrapper类,将一个函数作为参数传递给构造函数。该类的__call__方法被定义为执行传递的函数,并在执行前打印一条消息。然后,该函数被装饰器@Wrapper装饰,该装饰器实际上是实例化Wrapper类的语法糖。
在这个示例中,Wrapper类将add函数包装起来,并在执行前打印一条消息。当程序运行时,它将输出以下内容:
```
Function is being executed...
3
```
其中,“Function is being executed...”是我们添加的消息,而“3”是add函数的返回值。
相关问题
使用warpper类写一个java程序
### 回答1:
,实现从命令行读取文件路径和文件名,并将文件中的内容输出到控制台上。
答案:
您可以使用以下Java代码来实现:
import java.io.*;
public class FileWrapper {
public static void main(String[] args) {
String filePath = args[0];
String fileName = args[1];
try {
File file = new File(filePath, fileName);
BufferedReader br = new BufferedReader(new FileReader(file));
String line = br.readLine();
while (line != null) {
System.out.println(line);
line = br.readLine();
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
在命令行上,运行“java FileWrapper /path/to/file file.txt”就可以读取该文件并在控制台上输出其内容了。
### 回答2:
使用Wrapper类可以方便地将基本数据类型转换为对象,以便于进行一些特定的操作。下面是一个使用Wrapper类的Java程序示例。
首先,我们在程序中定义一个整数变量num,并赋值为10。然后,通过将num转换为Integer对象,我们可以使用Integer类提供的一些方法进行操作。例如,我们可以调用toString()方法将Integer对象转换为字符串,并使用length()方法获取字符串的长度。最后,我们再将字符串长度赋值给另一个整型变量length,并输出结果。
以下是具体的程序代码:
```java
public class WrapperExample {
public static void main(String[] args) {
int num = 10; // 定义一个整数变量num,赋值为10
Integer numObj = Integer.valueOf(num); // 将整数转换为Integer对象
String numStr = numObj.toString(); // 将Integer对象转换为字符串
int length = numStr.length(); // 获取字符串的长度
System.out.println("整数转换为字符串:" + numStr);
System.out.println("字符串长度为:" + length);
}
}
```
运行以上程序,将会输出如下结果:
```
整数转换为字符串:10
字符串长度为:2
```
通过上述程序示例,我们可以清楚地看到了如何使用Wrapper类将基本数据类型转换为对象,并利用Wrapper类提供的方法进行操作。这样,我们就可以更加灵活地处理基本数据类型了。
### 回答3:
使用wrapper类编写Java程序是一种常见的编程技巧,通过使用wrapper类可以轻松地进行一些常用的操作和功能。下面以一个计算器程序为例,展示如何使用wrapper类编写Java程序:
```java
public class Calculator {
public static void main(String[] args) {
Integer num1 = new Integer(10);
Integer num2 = new Integer(5);
// 进行加法运算
Integer sum = num1 + num2;
System.out.println("Sum: " + sum);
// 进行减法运算
Integer difference = num1 - num2;
System.out.println("Difference: " + difference);
// 进行乘法运算
Integer product = num1 * num2;
System.out.println("Product: " + product);
// 进行除法运算
Integer quotient = num1 / num2;
System.out.println("Quotient: " + quotient);
// 进行取模运算
Integer remainder = num1 % num2;
System.out.println("Remainder: " + remainder);
// 将包装类转换为基本数据类型
int n1 = num1.intValue();
int n2 = num2.intValue();
System.out.println("n1: " + n1 + ", n2: " + n2);
}
}
```
在这个例子中,我们使用了`Integer`这个wrapper类来进行计算。通过创建`Integer`对象,我们可以对其进行各种运算,如加法、减法、乘法、除法以及取模运算。此外,我们还可以通过`intValue()`方法将包装类转换为基本数据类型。
通过使用wrapper类,我们可以方便地进行数字的处理和运算,使得代码更加简洁和可读。
用warpper类写一个java小程序
好的,我能够帮你回答一些关于编程的问题。下面是一个使用wrapper类编写的Java小程序的基本框架:
```java
public class MyProgram {
public static void main(String[] args) {
// TODO: 在这里编写你的代码
// 示例:
Wrapper<String> stringWrapper = new Wrapper<>("Hello, World!");
System.out.println(stringWrapper.get());
}
}
class Wrapper<T> {
private T value;
public Wrapper(T value) {
this.value = value;
}
public T get() {
return value;
}
public void set(T value) {
this.value = value;
}
}
```
这个程序定义了一个`Wrapper`类,它用于包装一个任意类型的对象,并提供了getter和setter方法以访问包装的对象。`MyProgram`类中的`main`方法演示了如何使用这个类。
你可以根据自己的需求修改这个程序,添加更多的方法和实现特定的功能。希望这个基本框架能帮助到你。
阅读全文
相关推荐
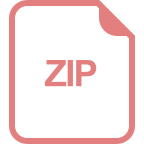
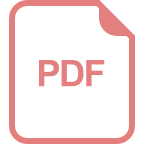


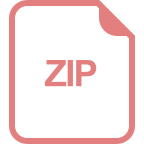
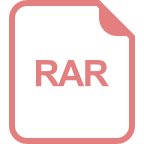
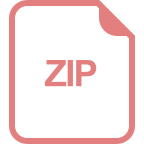
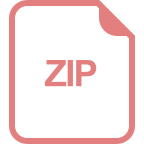
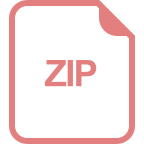
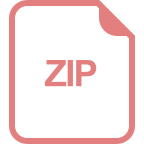
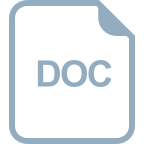
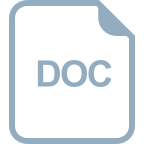



