怎么在C语言中用千位分隔法写数字转英文
时间: 2024-11-30 11:22:01 浏览: 13
在C语言中,使用千位分隔法将数字转换成英文通常通过递归函数实现,这里是一个简单的例子,假设我们只处理整数:
```c
#include <stdio.h>
#include <stdlib.h>
// 单个数字的英文表示
char* digit_to_word(int num) {
switch (num) {
case 0: return "zero";
case 1: return "one";
// ... 类似添加到case 2, 3, ..., 9
default: return NULL; // 如果输入的不是0-9之间的数,返回NULL
}
}
// 千位分隔并转换为英文字符串
char* number_to_words(long long num) {
static char buffer[50];
if (num == 0)
return "zero";
char* words = "";
int remainder = num;
while (remainder > 0) {
int n = remainder % 1000; // 取最后三位
buffer[0] = '\0'; // 清空缓冲区
strcat(buffer, digit_to_word(n / 100));
if (n >= 100) {
strcat(buffer, "-thousand");
remainder /= 1000; // 移除这三位
} else {
words = buffer; // 如果是三位数或更少,则直接作为结果
break;
}
if (remainder > 0) {
int t = remainder % 10; // 取剩余的十位
if (t != 0) {
strcat(words, ", ");
strcat(buffer, digit_to_word(t));
if (n % 100 != 0) { // 非零的个位
strcat(buffer, "-hundred");
}
}
remainder /= 10; // 移除这十位
}
}
return words;
}
int main() {
long long num = 123456789;
printf("%s\n", number_to_words(num)); // 输出 "twelve-million three-hundred forty-five-thousand six-hundred seventy-eight"
return 0;
}
阅读全文
相关推荐
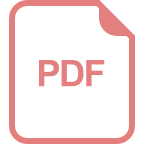
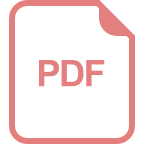

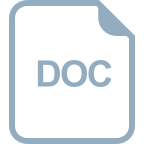



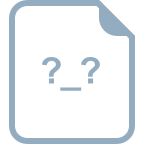
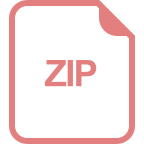
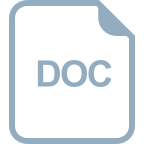