请编写一份详细代码,使用语言有Thymeleaf、Springboot,在Layui的动态的Table组件的表格单元格中,根据表格某一列的条件,1、获取指定某一列的进度对象,进行实时更新进度。2、根据进度,更新进度条背景颜色。
时间: 2024-04-16 19:28:56 浏览: 33
好的,以下是使用Thymeleaf和Spring Boot编写的代码示例:
在HTML模板中,使用Thymeleaf的语法来渲染动态的Table组件和进度条:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Dynamic Table with Progress Bar</title>
<!-- 导入Layui和自定义样式 -->
<link rel="stylesheet" href="https://cdn.staticfile.org/layui/2.5.6/css/layui.css">
<style>
.progress-bar {
width: 100%;
height: 20px;
background-color: #f2f2f2;
border-radius: 4px;
}
.progress-inner {
height: 100%;
border-radius: 4px;
}
</style>
</head>
<body>
<div class="layui-container">
<table class="layui-table">
<thead>
<tr>
<th>数据</th>
<th>进度</th>
</tr>
</thead>
<tbody>
<!-- 使用Thymeleaf循环渲染表格数据 -->
<tr th:each="data : ${datas}">
<td th:text="${data.name}"></td>
<td>
<div class="progress-bar">
<!-- 动态设置进度条背景颜色 -->
<div class="progress-inner" th:style="'width:' + ${data.progress} + '%; background-color:' + getProgressColor(data.progress)"></div>
</div>
</td>
</tr>
</tbody>
</table>
</div>
<!-- 导入Layui和自定义脚本 -->
<script src="https://cdn.staticfile.org/layui/2.5.6/layui.js"></script>
<script>
layui.use('table', function(){
var table = layui.table;
// 表格渲染
table.render({
elem: '.layui-table'
});
});
// 获取进度条背景颜色
function getProgressColor(progress) {
if (progress >= 80) {
return '#5FB878'; // 绿色
} else if (progress >= 50) {
return '#FFB800'; // 橙色
} else {
return '#FF5722'; // 红色
}
}
</script>
</body>
</html>
```
在Spring Boot的Controller中,使用Java代码来处理请求并返回动态表格的数据:
```java
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.ArrayList;
import java.util.List;
@Controller
@RequestMapping("/")
public class TableController {
@GetMapping("/table")
public String getTable(Model model) {
// 模拟从数据库或其他数据源获取表格数据
List<Data> datas = getDataFromDataSource();
model.addAttribute("datas", datas);
return "table";
}
private List<Data> getDataFromDataSource() {
// 模拟从数据库或其他数据源获取数据
List<Data> datas = new ArrayList<>();
datas.add(new Data("数据1", 60));
datas.add(new Data("数据2", 40));
datas.add(new Data("数据3", 90));
// ...
return datas;
}
// 数据模型类
public static class Data {
private String name;
private int progress;
public Data(String name, int progress) {
this.name = name;
this.progress = progress;
}
public String getName() {
return name;
}
public int getProgress() {
return progress;
}
}
}
```
以上代码通过Thymeleaf和Spring Boot实现了一个动态的Table组件,根据表格某一列的条件来实时更新进度和进度条的背景颜色。在HTML模板中使用Thymeleaf的语法循环渲染表格数据,并通过JavaScript函数动态设置进度条的宽度和背景颜色。在Spring Boot的Controller中,通过模拟从数据源获取表格数据,并将数据传递给HTML模板进行渲染。
请根据实际情况修改代码,并确保正确导入相关的依赖库。希望对你有所帮助!如有疑问,请随时追问。
相关推荐
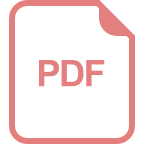
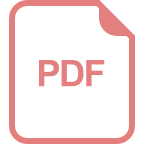














