满足一下要求写出代码网页中需要出现至少以下内容。 (1)、局部变量、全局变量的定义和使用 (2)、for…in、switch…case、if语句 (3)、至少一个函数的定义和调用 (4)、DOM对象属性和方法(/表示至少选做其中一个): 获得元素getElementById( )/ getElementByClassName( ) 对子节点的操作 appendChild(node)/removeChild(node)/replaceChild() 创建属性/元素/文本节点 createAttribute()/createElement()/createTextNode() 获得/设置属性getAttribute()/setAttribute() 属性innerHTML的使用 (5)、BOM对象属性和方法(/表示至少选做其中一个) 警告/确认/提示alert( )/confim( )/prompt( ) 定时器的使用setInterval( )/setTimeout( ) (6)、HTML5的全局方法 表单onchange/oninput onselect/onfocus/onblur 键盘onkeydown/onkeypress/onkeyup 鼠标onclick /ondblclick onmousedown/onmousemove/onmouseout /onmouseover/onmouseup 鼠标/键盘事件对象 client/client、screen/screenY、Location、 charCode/keyCode/which
时间: 2023-12-12 21:03:07 浏览: 88
以下是一个简单的示例代码,包含了问题中要求的内容:
```html
<!DOCTYPE html>
<html>
<head>
<title>示例代码</title>
<script>
// 定义全局变量
var globalVar = "Hello, world!";
window.onload = function() {
// 定义局部变量
var localVar = "This is a local variable.";
alert(localVar); // 警告框显示局部变量的值
// 使用 if 语句
if (globalVar.indexOf("Hello") !== -1) {
console.log("The global variable contains 'Hello'.");
}
// 使用 switch...case 语句
var num = 2;
switch (num) {
case 1:
console.log("The number is one.");
break;
case 2:
console.log("The number is two.");
break;
default:
console.log("The number is neither one nor two.");
}
// 使用 for...in 语句
var obj = {a: 1, b: 2, c: 3};
for (var prop in obj) {
console.log(prop + ": " + obj[prop]);
}
// 定义函数并调用
function myFunction() {
alert("This is my function.");
}
myFunction();
// 操作 DOM 对象
var elem = document.getElementById("myElement");
elem.innerHTML = "This is a new text.";
elem.setAttribute("class", "myClass");
var newElem = document.createElement("p");
newElem.innerHTML = "This is a new paragraph.";
elem.appendChild(newElem);
elem.removeChild(newElem);
// 使用 BOM 对象
var timerId = setTimeout(function() {
alert("This is a timeout function.");
}, 3000);
var result = confirm("Are you sure?");
if (result) {
location.href = "http://www.example.com";
}
// 使用 HTML5 全局方法
var inputElem = document.getElementById("myInput");
inputElem.oninput = function() {
console.log("The input value has changed.");
};
inputElem.onblur = function() {
console.log("The input has lost focus.");
};
var buttonElem = document.getElementById("myButton");
buttonElem.onclick = function() {
console.log("The button has been clicked.");
};
};
</script>
</head>
<body>
<input type="text" id="myInput">
<button id="myButton">Click me</button>
<div id="myElement">This is a div element.</div>
</body>
</html>
```
阅读全文
相关推荐
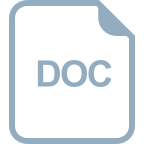
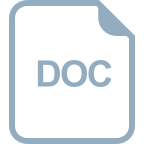
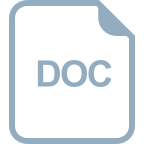
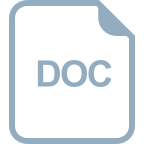
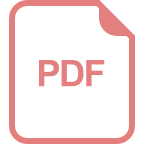
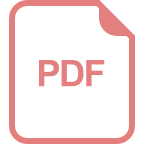
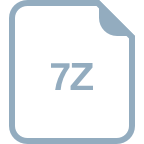
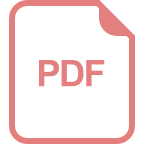
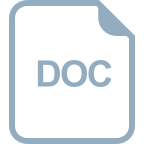
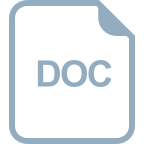
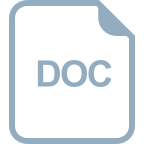
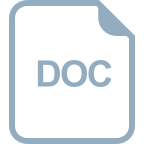
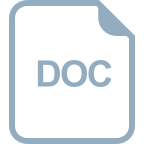
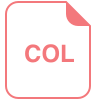
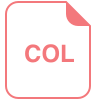
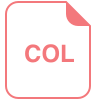


