TLC2543ADC用51写DIN输入DOUT输出低位先传
时间: 2023-05-30 16:01:50 浏览: 305
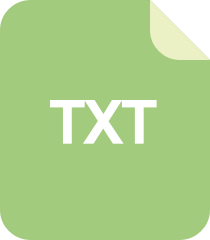
TLC2543的C51程序
下面是用51单片机编写TLC2543ADC的DIN输入、DOUT输出低位先传的代码:
```c
#include <reg51.h>
#define uchar unsigned char
#define uint unsigned int
sbit CLK = P3^3; // 时钟信号线
sbit CS = P3^4; // 片选信号线
sbit DOUT = P3^5; // 数据输出信号线
sbit DIN = P3^6; // 数据输入信号线
/* 延时函数,约为1us */
void Delay(uint count)
{
uint i;
for(i=0; i<count; i++);
}
/* 向TLC2543ADC发送1位数据 */
void SendBit(uchar bit)
{
DIN = bit; // 先将数据线传输需要发送的数据位
CLK = 0; // 将时钟线拉低
Delay(1); // 延时1us
CLK = 1; // 再将时钟线拉高,使TLC2543ADC读入数据
Delay(1); // 延时1us
}
/* 向TLC2543ADC发送1个字节的数据 */
void SendByte(uchar byte)
{
uchar i;
for(i=0; i<8; i++) // 依次发送8位数据
{
SendBit(byte & 0x01); // 发送最低位的数据位
byte >>= 1; // 将数据右移1位,准备发送下一位数据
}
}
/* 从TLC2543ADC读取1位数据 */
uchar ReadBit()
{
CLK = 0; // 将时钟线拉低
Delay(1); // 延时1us
CLK = 1; // 再将时钟线拉高,使TLC2543ADC输出数据
Delay(1); // 延时1us
return DOUT; // 读取数据线上的数据位
}
/* 从TLC2543ADC读取1个字节的数据 */
uchar ReadByte()
{
uchar i, byte=0;
for(i=0; i<8; i++) // 依次读取8位数据
{
byte >>= 1; // 将数据左移1位,为下一位数据位腾出位置
if(ReadBit()) // 如果读取到的数据位为1
{
byte |= 0x80; // 将最高位设置为1
}
}
return byte;
}
/* 初始化TLC2543ADC */
void InitTLC2543ADC()
{
CS = 1; // 先将片选线拉高
CLK = 0; // 将时钟线拉低
}
/* 读取TLC2543ADC的结果 */
uint ReadTLC2543ADC()
{
uchar i, byte1, byte2;
uint result;
CS = 0; // 将片选线拉低,开始传输数据
SendByte(0x80); // 发送起始字节
byte1 = ReadByte(); // 读取低8位数据
byte2 = ReadByte(); // 读取高8位数据
CS = 1; // 将片选线拉高,结束传输数据
result = byte1; // 将低8位数据存入结果变量中
result |= ((uint)byte2 << 8); // 将高8位数据左移8位,与低8位数据合并
return result;
}
/* 主函数 */
void main()
{
uint result;
InitTLC2543ADC(); // 初始化TLC2543ADC
while(1)
{
result = ReadTLC2543ADC(); // 读取TLC2543ADC的结果
// 这里可以对结果进行处理
}
}
```
上述代码中,`SendBit()`函数用于向TLC2543ADC发送1位数据,`SendByte()`函数用于向TLC2543ADC发送1个字节的数据,`ReadBit()`函数用于从TLC2543ADC读取1位数据,`ReadByte()`函数用于从TLC2543ADC读取1个字节的数据,`InitTLC2543ADC()`函数用于初始化TLC2543ADC,`ReadTLC2543ADC()`函数用于读取TLC2543ADC的结果。
在主函数中,先调用`InitTLC2543ADC()`函数初始化TLC2543ADC,然后进入循环读取TLC2543ADC的结果。读取到的结果可以根据实际需求进行处理。
阅读全文
相关推荐
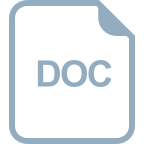
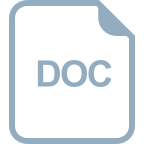





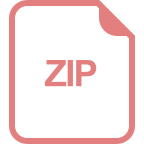
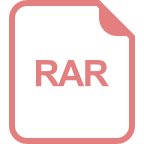
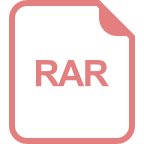
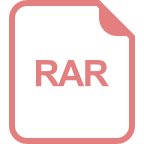
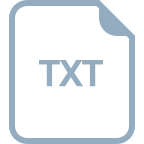
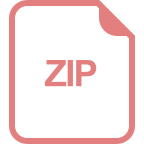
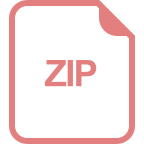


