用css,js写一个购物网站的的登陆界面,并且有验证码
时间: 2023-06-03 08:07:29 浏览: 73
很高兴回答您的问题!这是一个技术问题,我可以提供一些建议。首先,您可以使用HTML和CSS来设计登录页面,并使用JavaScript来验证表单。对于验证码的生成,您可以使用JavaScript和PHP等技术。您需要先设计验证码的外观,并给它一个随机的验证码值。之后,将验证码值与用户输入的值进行比较来验证用户的身份。如果需要更高级的验证码,您可以使用reCaptcha等技术。希望我的建议能够对您有所帮助!
相关问题
帮我写一个购物网站的登陆界面,用HTML和JavaScript
好的,以下是一个简单的购物网站登陆界面的HTML和JavaScript代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>购物网站登陆</title>
<meta charset="utf-8">
<style type="text/css">
form {
margin: 50px auto;
width: 400px;
text-align: center;
}
input {
padding: 10px;
margin: 10px;
border-radius: 5px;
border: none;
box-shadow: 1px 1px 3px gray;
}
button {
padding: 10px;
margin: 10px;
border-radius: 5px;
border: none;
background-color: blue;
color: white;
box-shadow: 1px 1px 3px gray;
cursor: pointer;
}
</style>
</head>
<body>
<form>
<h1>购物网站登陆</h1>
<input type="text" placeholder="用户名" id="username">
<input type="password" placeholder="密码" id="password">
<button type="button" onclick="login()">登陆</button>
</form>
<script type="text/javascript">
function login() {
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
if (username == "" || password == "") {
alert("用户名和密码不能为空!");
} else {
// 在这里写登陆验证的代码
// 如果验证通过,可以跳转到购物网站的主页
// window.location.href = "购物网站主页的URL";
}
}
</script>
</body>
</html>
```
这个界面包含一个表单,用户可以输入用户名和密码,点击“登陆”按钮后会调用login()函数进行登陆验证。在这个示例中,我们只是简单地检查了用户名和密码是否为空,如果为空则弹出一个提示框,否则可以在login()函数中编写登陆验证的代码。
注意,这个示例只是一个简单的登陆界面,实际的购物网站需要更多的功能和安全措施,例如验证码、密码加密、账户锁定等。
请用html+css帮我写一个登录界面需带有验证码
好的,以下是一个简单的带有验证码的登录页面示例,使用了HTML和CSS:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Page</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
}
.container {
width: 400px;
margin: 0 auto;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
padding: 20px;
box-sizing: border-box;
margin-top: 50px;
}
h2 {
text-align: center;
margin-top: 0;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
color: #333;
}
input[type=text], input[type=password], input[type=submit] {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: 1px solid #ccc;
border-radius: 3px;
box-sizing: border-box;
}
input[type=submit] {
background-color: #4CAF50;
color: #fff;
border: none;
cursor: pointer;
}
.captcha {
display: flex;
align-items: center;
justify-content: space-between;
margin-bottom: 20px;
}
.captcha-label {
flex: 1;
}
.captcha-img {
flex: 1;
margin-left: 20px;
}
.captcha-img img {
width: 100%;
border: 1px solid #ccc;
border-radius: 3px;
}
@media screen and (max-width: 480px) {
.container {
width: 90%;
}
}
</style>
</head>
<body>
<div class="container">
<h2>Login Page</h2>
<form action="#" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<div class="captcha">
<label class="captcha-label" for="captcha">Captcha:</label>
<div class="captcha-img">
<img src="generate_captcha.php" alt="Captcha Image">
</div>
</div>
<input type="text" id="captcha" name="captcha" required>
<input type="submit" value="Login">
</form>
</div>
</body>
</html>
```
在上面的示例中,我们使用了一个简单的表单来实现登录功能。其中,用户名和密码的输入框都添加了`required`属性,表示这两个字段是必填项。
同时,我们还添加了一个验证码输入框和一个验证码图片。验证码图片的URL指向`generate_captcha.php`,这是一个动态生成验证码图片的PHP脚本。当用户提交表单时,后端会通过验证输入的验证码是否和生成的一致,以确保用户是一个真实的人而不是机器人。
你可以通过以下CSS代码实现样式:
- `container`类定义了整个登录页面的样式,包括背景色、宽度、边框、内边距等。
- `h2`标签定义了标题的样式,使其居中显示并去掉默认的上边距。
- `label`标签定义了输入框前的标签的样式,包括加粗、颜色等。
- `input[type=text]`、`input[type=password]`和`input[type=submit]`定义了输入框和提交按钮的样式,包括宽度、内边距、边框、圆角等。
- `captcha`类定义了验证码输入框和验证码图片的样式,使用flex布局,让它们水平排列,并设置间距等。
- `captcha-label`类定义了验证码输入框前的标签的样式,使用flex布局,让它们占据相同的宽度。
- `captcha-img`类定义了验证码图片的样式,使用flex布局,让它占据剩余的宽度,并设置了左边距。
- `captcha-img img`定义了验证码图片的样式,设置了宽度、边框、圆角等。
希望以上内容能够帮助到你。
相关推荐
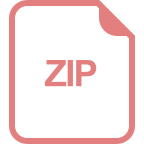
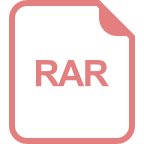
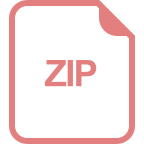












