3、用python输入一个字符串,判断字符串中有多少个字母?多少个数字?多少个其他符号?
时间: 2023-11-27 15:54:59 浏览: 103
可以使用 Python 中的 isalpha() 和 isdigit() 函数来判断字符串中的字母和数字,其他符号则可以通过判断字符是否既不是字母也不是数字来得到。具体实现如下:
```
string = input("请输入一个字符串:")
alpha_count = 0 # 统计字母个数
digit_count = 0 # 统计数字个数
other_count = 0 # 统计其他符号个数
for char in string:
if char.isalpha():
alpha_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("字母个数为:", alpha_count)
print("数字个数为:", digit_count)
print("其他符号个数为:", other_count)
```
运行程序后,输入一个字符串,程序会统计其中的字母、数字和其他符号的数量并输出。
相关问题
编写一个Python程序,要求能输入一个字符串,判断字符串中有多少个字母?多少个数字?多少个其他符号?
```
string = input("请输入一个字符串:")
letter_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("字符串中有{}个字母,{}个数字,{}个其他符号。".format(letter_count, digit_count, other_count))
```
注:isalpha()方法用于判断一个字符是否为字母,isdigit()方法用于判断一个字符是否为数字。
输入一个字符串,判断字符串中有多少个字母?多少个数字?多少个其他符号?
可以使用Python的字符串处理函数和正则表达式来实现。以下是一个示例代码:
```python
import re
s = input("请输入一个字符串:")
letter_count = 0
digit_count = 0
other_count = 0
for c in s:
if c.isalpha():
letter_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
# 也可以使用正则表达式来实现
# letter_count = len(re.findall(r'[a-zA-Z]', s))
# digit_count = len(re.findall(r'\d', s))
# other_count = len(re.findall(r'[^a-zA-Z\d]', s))
print("字母数量:", letter_count)
print("数字数量:", digit_count)
print("其他符号数量:", other_count)
```
示例输出:
```
请输入一个字符串:Hello, world! 123.
字母数量: 10
数字数量: 3
其他符号数量: 4
```
阅读全文
相关推荐
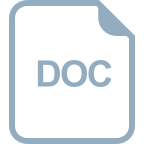
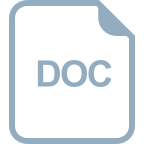
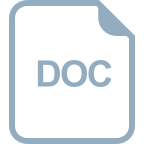













