上述提到的pcl::KMeans详细实现代码
时间: 2024-03-20 11:44:55 浏览: 83
下面是一个简单的使用pcl::KMeans实现k-means聚类的示例代码:
```c++
#include <pcl/features/normal_3d.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/kdtree/kdtree_flann.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/features/fpfh.h>
#include <pcl/kdtree/kmeans.h>
// 定义点云类型
typedef pcl::PointXYZ PointType;
typedef pcl::PointCloud<PointType> PointCloud;
typedef pcl::Normal NormalType;
typedef pcl::PointCloud<NormalType> NormalCloud;
typedef pcl::FPFHSignature33 FeatureType;
typedef pcl::PointCloud<FeatureType> FeatureCloud;
int main()
{
// 加载点云数据
PointCloud::Ptr cloud(new PointCloud);
pcl::io::loadPCDFile("input.pcd", *cloud);
// 下采样
pcl::VoxelGrid<PointType> grid;
grid.setLeafSize(0.01, 0.01, 0.01);
grid.setInputCloud(cloud);
PointCloud::Ptr cloud_downsampled(new PointCloud);
grid.filter(*cloud_downsampled);
// 计算法线
pcl::NormalEstimation<PointType, NormalType> ne;
ne.setInputCloud(cloud_downsampled);
pcl::search::KdTree<PointType>::Ptr tree(new pcl::search::KdTree<PointType>);
ne.setSearchMethod(tree);
NormalCloud::Ptr normals(new NormalCloud);
ne.setRadiusSearch(0.03);
ne.compute(*normals);
// 计算特征
pcl::FPFHEstimation<PointType, NormalType, FeatureType> fpfh;
fpfh.setInputCloud(cloud_downsampled);
fpfh.setInputNormals(normals);
fpfh.setSearchMethod(tree);
FeatureCloud::Ptr features(new FeatureCloud);
fpfh.setRadiusSearch(0.05);
fpfh.compute(*features);
// k-means聚类
int k = 3;
pcl::KMeans<FeatureType> kmeans;
kmeans.setClusterSize(k);
kmeans.setEuclideanDistance(true);
kmeans.setInputCloud(features);
std::vector<pcl::PointIndices> cluster_indices;
kmeans.extract(cluster_indices);
// 输出聚类结果
for (int i = 0; i < cluster_indices.size(); i++)
{
std::cout << "Cluster " << i << ":\n";
for (int j = 0; j < cluster_indices[i].indices.size(); j++)
{
std::cout << cluster_indices[i].indices[j] << " ";
}
std::cout << std::endl;
}
return 0;
}
```
这段代码首先加载了一个点云数据,然后进行下采样、计算法线和特征等操作。接着使用pcl::KMeans对特征向量进行聚类,得到聚类结果。最后将聚类结果输出到控制台中。需要注意的是这里的特征类型是pcl::FPFHSignature33,如果使用其他类型的特征,需要将pcl::KMeans的模板参数替换成对应的类型。
阅读全文
相关推荐
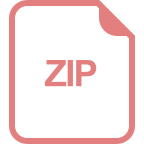
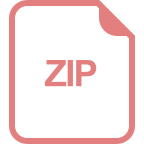
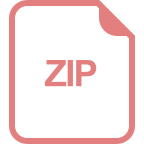

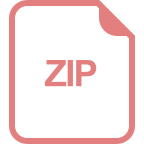
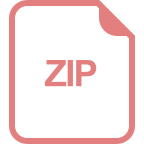
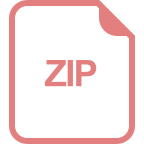
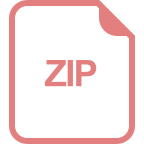
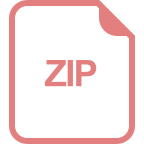
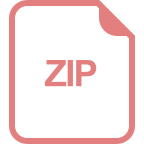
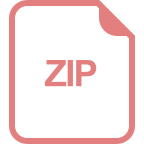
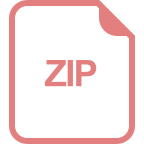
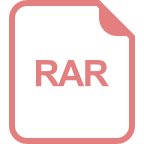
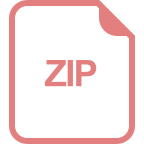
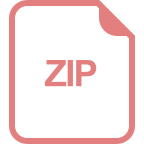
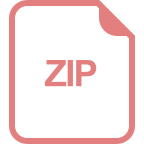
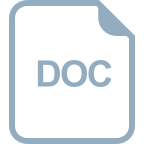
