python学生管理系统代码及其界面
时间: 2023-09-03 10:08:49 浏览: 142
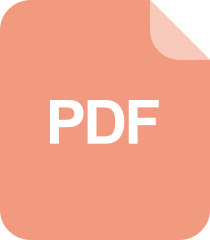
python学生信息管理系统(初级版)

这里提供一个简单的Python学生管理系统代码及其界面。请注意,这是一个基于命令行的系统,没有图形用户界面。
代码:
```python
class Student:
def __init__(self, name, age, grade):
self.name = name
self.age = age
self.grade = grade
class StudentManager:
def __init__(self):
self.students = []
def add_student(self, student):
self.students.append(student)
def remove_student(self, name):
for student in self.students:
if student.name == name:
self.students.remove(student)
return True
return False
def print_students(self):
for student in self.students:
print(f"Name: {student.name}, Age: {student.age}, Grade: {student.grade}")
manager = StudentManager()
while True:
print("\nWelcome to the Student Management System")
print("1. Add Student")
print("2. Remove Student")
print("3. View Students")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == "1":
name = input("Enter the student's name: ")
age = int(input("Enter the student's age: "))
grade = int(input("Enter the student's grade: "))
student = Student(name, age, grade)
manager.add_student(student)
print(f"{name} added to the system.")
elif choice == "2":
name = input("Enter the student's name: ")
if manager.remove_student(name):
print(f"{name} removed from the system.")
else:
print(f"{name} not found in the system.")
elif choice == "3":
manager.print_students()
elif choice == "4":
print("Thank you for using the Student Management System.")
break
else:
print("Invalid choice. Please try again.")
```
界面:
```
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 1
Enter the student's name: John
Enter the student's age: 18
Enter the student's grade: 12
John added to the system.
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 1
Enter the student's name: Mary
Enter the student's age: 17
Enter the student's grade: 11
Mary added to the system.
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 3
Name: John, Age: 18, Grade: 12
Name: Mary, Age: 17, Grade: 11
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 2
Enter the student's name: John
John removed from the system.
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 3
Name: Mary, Age: 17, Grade: 11
Welcome to the Student Management System
1. Add Student
2. Remove Student
3. View Students
4. Exit
Enter your choice: 4
Thank you for using the Student Management System.
```
阅读全文
相关推荐
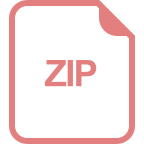
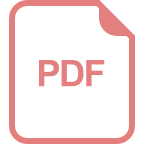

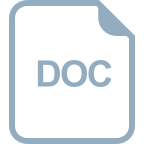
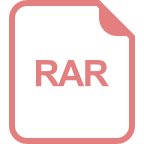
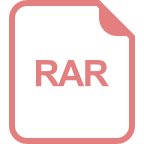
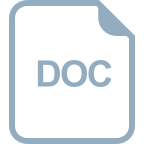
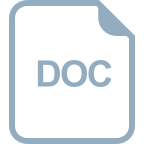
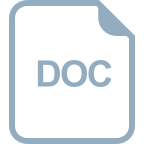
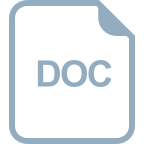
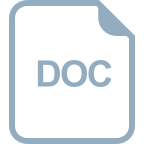
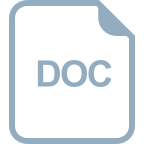
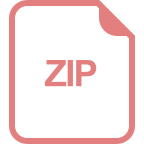
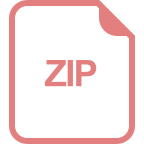
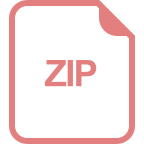
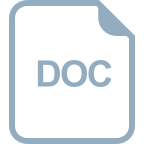
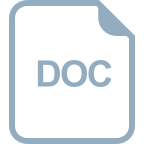