在MySort.cpp中完成三个排序方法。 bubbleSort(int* arr, int len); 实现冒泡排序,需要排序的数组为arr,数组长度为len quickSort(int* arr, int len); 实现快速排序 heapSort(int* arr, int len); 实现堆排序
时间: 2023-08-28 14:21:39 浏览: 44
好的,以下是 MySort.cpp 中三个排序方法的实现:
1. 冒泡排序:
```cpp
void bubbleSort(int* arr, int len) {
for (int i = 0; i < len - 1; i++) {
for (int j = 0; j < len - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
2. 快速排序:
```cpp
void quickSort(int* arr, int len) {
if (len <= 1) return;
int pivot = arr[0];
int left = 0, right = len - 1;
while (left < right) {
while (left < right && arr[right] >= pivot) right--;
arr[left] = arr[right];
while (left < right && arr[left] <= pivot) left++;
arr[right] = arr[left];
}
arr[left] = pivot;
quickSort(arr, left);
quickSort(arr + left + 1, len - left - 1);
}
```
3. 堆排序:
```cpp
void heapSort(int* arr, int len) {
for (int i = len / 2 - 1; i >= 0; i--) {
heapify(arr, len, i);
}
for (int i = len - 1; i >= 0; i--) {
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
heapify(arr, i, 0);
}
}
void heapify(int* arr, int len, int root) {
int largest = root;
int left = 2 * root + 1;
int right = 2 * root + 2;
if (left < len && arr[left] > arr[largest]) {
largest = left;
}
if (right < len && arr[right] > arr[largest]) {
largest = right;
}
if (largest != root) {
int temp = arr[root];
arr[root] = arr[largest];
arr[largest] = temp;
heapify(arr, len, largest);
}
}
```
需要注意的是,这些排序方法都有其优缺点,对于不同的数据集和应用场景,应该选择不同的排序方法来获得更好的性能。同时,在实际开发中,也可以使用标准库中的排序算法,例如 std::sort()。
相关推荐
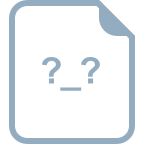
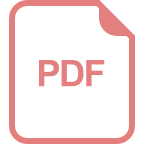
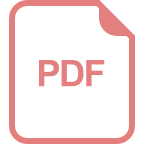














