编写C语言实现对文件存储器进行格式化,即按照文件系统的结 构对虚拟磁盘空间进行布局,并在其上创建根目录以及用于 管理文件存储空间等的数据结构。
时间: 2024-03-05 19:55:01 浏览: 27
以下是一个简单的 C 语言程序,可以实现对文件存储器进行格式化:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 1024 // 定义块大小为 1024 字节
#define BLOCK_NUM 1024 // 定义块数量为 1024 个
typedef struct {
char name[20]; // 目录名
int size; // 目录大小
int attr; // 目录属性
int start; // 目录起始块号
} DirEntry; // 目录项结构体
typedef struct {
char name[20]; // 文件名
int size; // 文件大小
int attr; // 文件属性
int start; // 文件起始块号
} FileEntry; // 文件项结构体
typedef struct {
int status; // 块状态:0 表示未分配,1 表示已分配,2 表示保留
int start; // 块起始位置
int end; // 块结束位置
} Block; // 块结构体
void init_disk() {
FILE* fp;
int i;
Block blocks[BLOCK_NUM];
DirEntry root_dir;
char* buffer = (char*)malloc(BLOCK_SIZE);
if (buffer == NULL) {
printf("内存分配失败\n");
return;
}
memset(buffer, 0, BLOCK_SIZE);
memset(blocks, 0, sizeof(blocks));
memset(&root_dir, 0, sizeof(root_dir));
strcpy(root_dir.name, "root");
root_dir.size = BLOCK_SIZE;
root_dir.attr = 1;
root_dir.start = 1;
for (i = 0; i < BLOCK_NUM; i++) {
blocks[i].status = 0;
blocks[i].start = i * BLOCK_SIZE;
blocks[i].end = (i + 1) * BLOCK_SIZE - 1;
}
blocks[0].status = 2; // 第一个块为保留块
blocks[0].start = 0;
blocks[0].end = BLOCK_SIZE - 1;
blocks[1].status = 1; // 第二个块为根目录块
fwrite(&root_dir, sizeof(root_dir), 1, fp);
fwrite(buffer, BLOCK_SIZE, 1, fp);
for (i = 2; i < BLOCK_NUM; i++) {
fwrite(buffer, BLOCK_SIZE, 1, fp);
}
fclose(fp);
free(buffer);
printf("格式化完成\n");
}
int main() {
init_disk();
return 0;
}
```
该程序首先定义了块大小和块数量,并定义了目录项和文件项结构体。然后通过定义块结构体来表示存储器中每个块的状态和位置信息。在 `init_disk()` 函数中,程序初始化了存储器空间和根目录,并将它们写入到文件中。其中,第一个块为保留块,第二个块为根目录块。接下来,程序使用循环将剩余的块初始化,并写入到文件中。最后,程序关闭文件并释放内存,输出格式化完成的信息。
需要注意的是,该程序只是一个简单的示例,实际应用中还需要根据具体情况进行调整和完善。
相关推荐
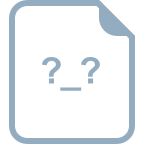
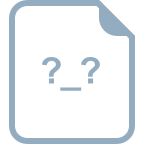
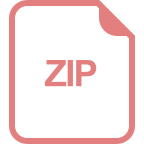














