抓取国家电网电子商务平台所有招标公告,下载项目公告文件,代码示例
时间: 2024-03-29 07:41:21 浏览: 138
抓取国家电网电子商务平台所有招标公告并下载项目公告文件可以使用 Python 网络爬虫实现。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import os
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 获取页面内容的函数
def get_html(url):
response = requests.get(url, headers=headers)
response.encoding = 'utf-8'
html = response.text
return html
# 获取所有招标公告的链接
def get_links():
links = []
for page in range(1, 3): # 暂时只爬前两页
url = f'http://ecp.sgcc.com.cn/ecp2.0/ecp/search/notice.jsp?page={page}¬ice_type=2'
html = get_html(url)
soup = BeautifulSoup(html, 'html.parser')
notices = soup.select('.m_m_c_list tr')
for notice in notices:
link = notice.select_one('a')['href']
links.append(link)
return links
# 下载项目公告文件
def download_files():
links = get_links()
for link in links:
url = f'http://ecp.sgcc.com.cn{link}'
html = get_html(url)
soup = BeautifulSoup(html, 'html.parser')
title = soup.select_one('.detail_tit').get_text()
file_links = soup.select('.m2 a')
for file_link in file_links:
file_url = f'http://ecp.sgcc.com.cn{file_link["href"]}'
file_name = file_link.get_text()
# 创建文件夹
if not os.path.exists(title):
os.makedirs(title)
# 下载文件
with open(f'{title}/{file_name}', 'wb') as f:
f.write(requests.get(file_url).content)
if __name__ == '__main__':
download_files()
```
这个示例代码使用了 requests 库和 BeautifulSoup 库,分别用于发送网络请求和解析 HTML 页面。首先使用 get_links 函数获取所有招标公告的链接,然后遍历每个链接,使用 download_files 函数下载项目公告文件。在下载文件之前,需要先创建相应的目录。
阅读全文
相关推荐
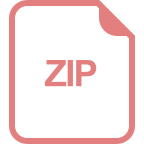
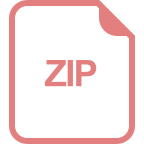
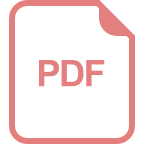
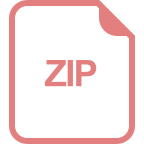
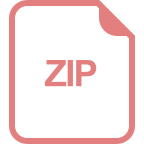
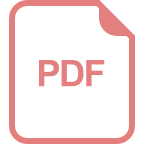
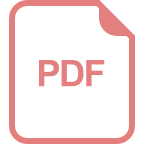
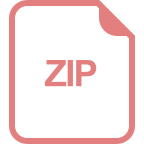
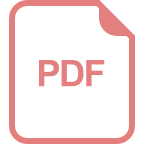
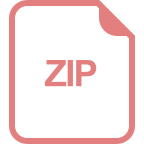
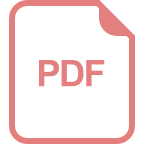
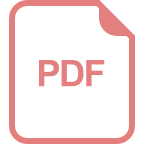
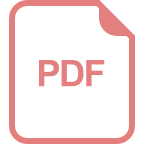
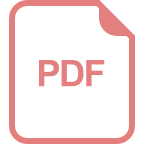
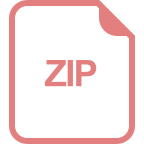
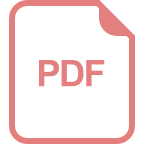