Implement a function that equalizes grey-scale images based on their histogram. The input is a given grey level image; the output is the derived image with uniform intensity distribution.
时间: 2024-03-03 19:50:21 浏览: 130
Sure, I can help you with that! Here is a Python implementation of the function:
```python
import numpy as np
import cv2
def hist_equalization(img):
# Calculate histogram
hist, bins = np.histogram(img.flatten(), 256, [0, 256])
# Calculate cumulative distribution function (CDF)
cdf = hist.cumsum()
# Normalize CDF
cdf_normalized = cdf * hist.max() / cdf.max()
# Create lookup table
lut = np.interp(np.arange(256), bins[:-1], cdf_normalized)
# Apply lookup table to image
equalized_img = cv2.LUT(img, lut)
return equalized_img
```
To use this function, simply pass in a grayscale image as the argument and it will return the equalized image. Here's an example of how to use it:
```python
# Load grayscale image
img = cv2.imread('image.jpg', 0)
# Apply histogram equalization
equalized_img = hist_equalization(img)
# Display original and equalized images
cv2.imshow('Original Image', img)
cv2.imshow('Equalized Image', equalized_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
I hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
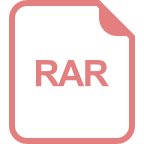














