std::tuple获取值
时间: 2023-07-28 14:53:11 浏览: 56
要获取 `std::tuple` 中的值,可以使用 `std::get` 函数。这个函数的第一个参数是 `std::tuple` 对象,第二个参数是要获取的元素的索引。例如,假设有一个 `std::tuple<int, double, std::string>` 对象,要获取第二个元素(即 `double` 类型),可以这样写:
```c++
#include <tuple>
#include <iostream>
int main() {
std::tuple<int, double, std::string> my_tuple(42, 3.14, "hello");
double my_double = std::get<1>(my_tuple);
std::cout << my_double << std::endl;
return 0;
}
```
输出:
```
3.14
```
相关问题
cpp11std::tuple 和 std::make_tuple
cpp11std::tuple 和 std::make_tuple 是 C++11 引入的两个新特性。
cpp11std::tuple 是一个用于存储多个值的容器,类似于 std::pair,但是可以存储多个值。cpp11std::tuple 在定义时需要指定每个元素的类型,并且可以通过 std::get 函数来获取每个元素的值。
std::make_tuple 是一个用于创建 cpp11std::tuple 对象的函数模板。它可以接受任意数量的参数,并将这些参数打包为一个 cpp11std::tuple 对象返回。
例如:
```
#include <tuple>
#include <string>
#include <iostream>
int main()
{
cpp11std::tuple<int, std::string, double> t(42, "hello", 3.14);
std::cout << std::get<0>(t) << " " << std::get<1>(t) << " " << std::get<2>(t) << std::endl;
auto t2 = std::make_tuple(42, "world", 2.71);
std::cout << std::get<0>(t2) << " " << std::get<1>(t2) << " " << std::get<2>(t2) << std::endl;
}
```
输出:
```
42 hello 3.14
42 world 2.71
```
其中,第一个例子是直接定义 cpp11std::tuple 对象,第二个例子使用了 std::make_tuple 函数来创建 cpp11std::tuple 对象。
std::tuple源码
对于std::tuple的源码,我无法直接提供给你。std::tuple是C++标准库中的一部分,其源码由C++标准库的实现者提供和维护。你可以查找你所使用的C++编译器的标准库实现的源代码,以了解std::tuple的实现细节。
然而,我可以告诉你一些关于std::tuple的基本知识。std::tuple是一个模板类,它可以容纳多个不同类型的值,并提供了一组操作函数,用于访问和操作这些值。通过使用std::tuple,可以将多个不同类型的值打包在一起,这在某些情况下非常有用。
std::tuple的大小是在编译时确定的,并且可以使用std::tuple_size模板函数来获取tuple的参数个数。可以使用std::get函数来获取tuple中的任意元素的值。
需要注意的是,std::tuple的索引是从0开始的,因此要访问第一个元素,可以使用std::get<0>(tuple)。
希望这些信息对你有帮助。如果你需要更多关于std::tuple的详细信息,我建议你查阅C++标准库的相关文档或参考资料。
相关推荐
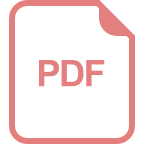
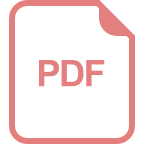
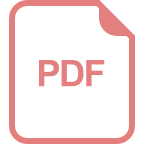












