input_img = torch.cat([img, mask], dim=1)
时间: 2024-06-05 13:11:33 浏览: 8
这行代码使用 PyTorch 中的 `torch.cat` 函数将 `img` 和 `mask` 沿着第一个维度(即通道维度)拼接起来,生成一个新的张量 `input_img`。这里假设 `img` 和 `mask` 的形状都是 `[batch_size, channel, height, width]`,那么拼接后的 `input_img` 的形状就是 `[batch_size, channel*2, height, width]`。这种拼接操作通常用于将多个输入数据(比如图像和掩膜)合并成一个输入。
相关问题
combined_node_feat = torch.cat((old_node_feat, new_node_feat), dim=1)
这行代码使用 PyTorch 中的 `torch.cat` 函数将两个张量 `old_node_feat` 和 `new_node_feat` 沿着第二个维度(即列)拼接在一起,生成一个新的张量 `combined_node_feat`。
假设 `old_node_feat` 的形状为 (n, m1),`new_node_feat` 的形状为 (n, m2),那么 `combined_node_feat` 的形状将会是 (n, m1+m2)。
具体实现可以看下面的示例代码:
```python
import torch
# 假设 old_node_feat 和 new_node_feat 是两个张量
old_node_feat = torch.randn(10, 5) # 形状为 (10, 5) 的张量
new_node_feat = torch.randn(10, 3) # 形状为 (10, 3) 的张量
# 使用 torch.cat 将两个张量拼接在一起
combined_node_feat = torch.cat((old_node_feat, new_node_feat), dim=1) # 沿着第二个维度拼接
# 打印出新的张量的形状
print(combined_node_feat.shape) # 输出 (10, 8)
```
这里将 `old_node_feat` 和 `new_node_feat` 沿着第二个维度拼接在一起,生成了一个形状为 (10, 8) 的新张量 `combined_node_feat`。
解释代码contrast_feature = torch.cat(torch.unbind(features, dim=1), dim=0)
代码`contrast_feature = torch.cat(torch.unbind(features, dim=1 dim=0)`的作用是将维度为1的特征张量进行拼接,并将结果保存在`contrast_feature`中。
首先,`torch.unbind(features, dim=1)`将张量`features`在维度1上进行拆分,返回一个元组。这个元组包含了在维度1上切分的多个子张量。
然后,`torch.cat`函数接受一个可迭代对象作为输入,并沿指定的维度进行拼接。在这里,我们将通过`torch.unbind`得到的多个子张量作为可迭代对象传递给`torch.cat`函数,并指定`dim=0`表示在维度0上进行拼接。
最终,拼接的结果被赋值给变量`contrast_feature`,它将包含原始特征张量中所有维度为1的子张量按顺序拼接在一起的结果。
举个例子,假设原始特征张量`features`的形状为`(3, 4, 2)`,其中3表示样本数,4表示特征数,2表示维度1的大小。那么通过执行上述代码,`contrast_feature`将是一个形状为`(12, 2)`的张量,其中12是样本数和特征数的乘积,2是维度1的大小。
相关推荐
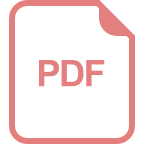
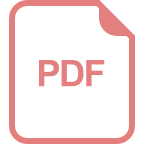












