c语言代码 设有学生信息如下:学号(长整型)、姓名(字符串型)、年龄(整型)、课程1成绩(实型)、课程2成绩(实型)、课程3成绩(实型)、课程4成绩(实型)、课程5成绩(实型)、课程6成绩(实型)、课程6成绩(实型)、总分(实型)、平均分(实型)。试编写程序,输入3个学生的上述信息,计算每个学生的总分、平均分,然后输出每个学生学号、姓名、总分、平均分。
时间: 2023-07-04 11:04:50 浏览: 107
以下是使用结构体和数组存储学生信息,计算总分和平均分,最后输出结果的 c 代码:
```c
#include <stdio.h>
struct student {
long int number; // 学号
char name[20]; // 姓名
int age; // 年龄
float score[6]; // 课程成绩
float total; // 总分
float average; // 平均分
};
int main() {
struct student stu[3];
int i, j;
// 输入每个学生的信息和成绩
for(i = 0; i < 3; i++) {
printf("请输入第%d个学生的信息:\n", i+1);
printf("学号:"); scanf("%ld", &stu[i].number);
printf("姓名:"); scanf("%s", &stu[i].name);
printf("年龄:"); scanf("%d", &stu[i].age);
for(j = 0; j < 6; j++) {
printf("请输入第%d门课的成绩:", j+1);
scanf("%f", &stu[i].score[j]);
}
printf("\n");
}
// 计算每个学生的总分和平均分
for(i = 0; i < 3; i++) {
stu[i].total = 0;
for(j = 0; j < 6; j++) {
stu[i].total += stu[i].score[j];
}
stu[i].average = stu[i].total / 6.0;
}
// 输出结果
printf("学号\t姓名\t总分\t平均分\n");
for(i = 0; i < 3; i++) {
printf("%ld\t%s\t%.2f\t%.2f\n", stu[i].number, stu[i].name, stu[i].total, stu[i].average);
}
return 0;
}
```
注意,以上代码中使用了数组存储 3 个学生的信息,因此输入时需要循环 3 次,计算总分和平均分时也需要循环 3 次。输出时,学生的信息和成绩需要一一对应输出。
相关推荐
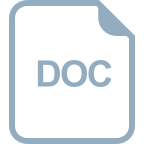
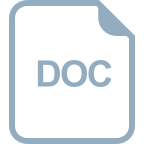
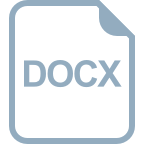
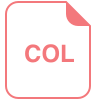
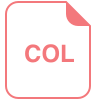
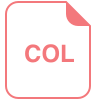
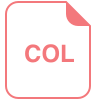
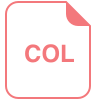











