unity加载streamingassets下的ply模型并显示到场景中
时间: 2024-03-28 12:37:51 浏览: 23
在Unity中,可以使用以下代码加载StreamingAssets文件夹中的PLY模型并显示到场景中:
```csharp
// 获取StreamingAssets文件夹的路径
string streamingAssetsPath = Application.streamingAssetsPath;
// 加载PLY模型
string filePath = Path.Combine(streamingAssetsPath, "example.ply");
StreamReader reader = new StreamReader(filePath);
string contents = reader.ReadToEnd();
reader.Close();
// 解析PLY模型数据
string[] lines = contents.Split('\n');
int vertexCount = 0;
List<Vector3> vertices = new List<Vector3>();
List<int> indices = new List<int>();
for (int i = 0; i < lines.Length; i++) {
string line = lines[i].Trim();
if (line.StartsWith("element vertex")) {
vertexCount = int.Parse(line.Split(' ')[2]);
} else if (line.StartsWith("end_header")) {
// 读取顶点数据
for (int j = 0; j < vertexCount; j++) {
string vertexLine = lines[i + j + 1].Trim();
string[] vertexValues = vertexLine.Split(' ');
Vector3 vertex = new Vector3(float.Parse(vertexValues[0]), float.Parse(vertexValues[1]), float.Parse(vertexValues[2]));
vertices.Add(vertex);
}
// 读取索引数据
for (int j = vertexCount + 1; j < lines.Length - i - 1; j++) {
string indexLine = lines[i + j + 1].Trim();
string[] indexValues = indexLine.Split(' ');
indices.Add(int.Parse(indexValues[1]));
indices.Add(int.Parse(indexValues[2]));
indices.Add(int.Parse(indexValues[3]));
}
break;
}
}
// 创建Mesh对象
Mesh mesh = new Mesh();
mesh.SetVertices(vertices);
mesh.SetIndices(indices.ToArray(), MeshTopology.Triangles, 0);
// 创建GameObject并添加MeshRenderer和MeshFilter组件
GameObject obj = new GameObject();
obj.AddComponent<MeshRenderer>();
obj.AddComponent<MeshFilter>();
obj.GetComponent<MeshFilter>().mesh = mesh;
// 将GameObject添加到场景中
SceneManager.MoveGameObjectToScene(obj, SceneManager.GetActiveScene());
```
需要注意的是,这个代码只能加载简单的PLY模型,如果需要加载复杂的PLY模型,可能需要使用一些开源的PLY解析器库。另外,由于Unity使用左手坐标系,而PLY格式使用右手坐标系,因此在加载PLY模型之后,需要将其坐标系进行转换。
相关推荐
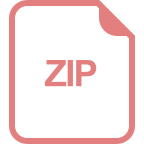
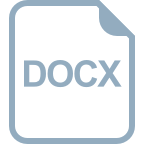














