mybatis 返回Integer数组
时间: 2023-07-17 20:56:09 浏览: 161
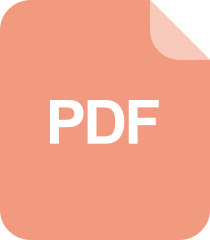
Mybatis返回int或者Integer类型报错的解决办法

在 MyBatis 中,可以使用 ResultMap 来映射查询结果到 Java 对象。如果你想要返回一个 Integer 数组,可以按照以下步骤操作:
1. 首先,在你的 Mapper XML 文件中定义一个 ResultMap,指定返回类型为 Integer 数组:
```xml
<resultMap id="integerArrayResultMap" type="java.lang.Integer" >
<id property="id" column="id" />
<!-- 根据你的数据库表结构定义其他属性映射 -->
</resultMap>
```
2. 然后,在对应的查询语句中使用该 ResultMap:
```xml
<select id="getIntegerArray" resultMap="integerArrayResultMap">
SELECT id FROM your_table
</select>
```
3. 最后,在对应的 Mapper 接口中定义方法来执行该查询:
```java
@Select("getIntegerArray")
Integer[] getIntegerArray();
```
这样,在调用 `getIntegerArray()` 方法时,MyBatis 会执行对应的 SQL 查询,并将结果映射为一个 Integer 数组返回给你。
阅读全文
相关推荐
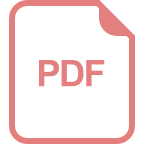


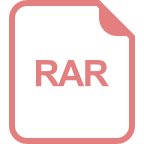
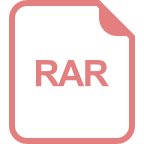
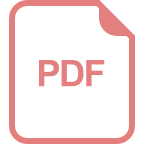
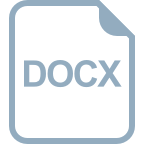
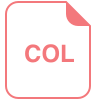
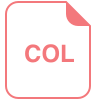
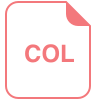






