用java实现结合GUI程序设计,编写选号程序,要求在窗体中安排6个标签,每个标签上显示0~9之间的一位数字,每位数字用一个线程控制其变化,单击“停止”按钮则所有标签数字停止变化。
时间: 2024-03-02 13:50:29 浏览: 81
好的,这是一个比较有趣的问题。首先,我们需要创建一个 Java GUI 应用程序,并在窗体中添加6个标签和一个“停止”按钮。然后,我们需要编写6个线程,每个线程控制一个标签上的数字变化。最后,我们需要在“停止”按钮上添加一个事件监听器,以便在单击按钮时停止所有线程。
下面是实现这个程序的详细步骤:
1. 创建一个 Java GUI 应用程序。
我们可以使用 Java Swing 框架来创建 GUI 应用程序。我们需要创建一个 JFrame 对象,并在其中添加6个标签和一个“停止”按钮。代码如下:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class NumberPicker extends JFrame {
private JLabel[] labels = new JLabel[6];
private JButton stopButton;
public NumberPicker() {
setTitle("选号程序");
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(2, 6));
for (int i = 0; i < 6; i++) {
labels[i] = new JLabel("0", JLabel.CENTER);
labels[i].setFont(new Font("Courier New", Font.BOLD, 48));
add(labels[i]);
}
stopButton = new JButton("停止");
stopButton.setFont(new Font("Courier New", Font.BOLD, 24));
stopButton.addActionListener(new StopButtonListener());
add(stopButton);
setVisible(true);
}
private class StopButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
// TODO: 停止所有线程
}
}
public static void main(String[] args) {
new NumberPicker();
}
}
```
2. 编写6个线程控制标签上的数字变化。
我们需要编写6个线程,每个线程控制一个标签上的数字变化。为了方便起见,我们可以将所有线程都定义为内部类,并让它们继承 Thread 类。在每个线程的 run() 方法中,我们需要使用 Thread.sleep() 方法来控制数字变化的速度。代码如下:
```
private class NumberThread extends Thread {
private JLabel label;
public NumberThread(JLabel label) {
this.label = label;
}
public void run() {
while (!isInterrupted()) {
int number = (int) (Math.random() * 10);
label.setText(String.valueOf(number));
try {
Thread.sleep(100);
} catch (InterruptedException e) {
interrupt();
}
}
}
}
for (int i = 0; i < 6; i++) {
NumberThread thread = new NumberThread(labels[i]);
thread.start();
}
```
3. 在“停止”按钮上添加事件监听器。
最后,我们需要在“停止”按钮上添加一个事件监听器,以便在单击按钮时停止所有线程。我们可以在 StopButtonListener 内部类中定义一个 stop() 方法,该方法会遍历所有线程并调用其 interrupt() 方法。代码如下:
```
private class StopButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
for (int i = 0; i < 6; i++) {
Thread thread = labels[i].getThread();
if (thread != null) {
thread.interrupt();
}
}
}
}
```
完整代码如下:
阅读全文
相关推荐




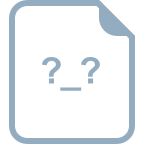







