利用pyspark做决策树代码
时间: 2024-03-22 08:18:52 浏览: 51
下面是一个简单的 pyspark 决策树代码,用于分类任务:
```python
from pyspark.ml import Pipeline
from pyspark.ml.feature import VectorAssembler, StringIndexer
from pyspark.ml.classification import DecisionTreeClassifier
from pyspark.ml.evaluation import MulticlassClassificationEvaluator
# 读取数据
data = spark.read.csv('path/to/data.csv', header=True, inferSchema=True)
# 特征工程
assembler = VectorAssembler(inputCols=['feature1', 'feature2', 'feature3'], outputCol='features')
labelIndexer = StringIndexer(inputCol='label', outputCol='indexedLabel')
# 拆分数据集为训练集和测试集
(trainData, testData) = data.randomSplit([0.7, 0.3], seed=123)
# 建立决策树模型
dt = DecisionTreeClassifier(labelCol='indexedLabel', featuresCol='features')
# 建立模型管道
pipeline = Pipeline(stages=[assembler, labelIndexer, dt])
# 训练模型
model = pipeline.fit(trainData)
# 测试模型
predictions = model.transform(testData)
# 评估模型
evaluator = MulticlassClassificationEvaluator(labelCol='indexedLabel', predictionCol='prediction', metricName='accuracy')
accuracy = evaluator.evaluate(predictions)
print('Test Accuracy = %g' % accuracy)
```
这个示例代码假设你有一个 CSV 数据文件,其中包含三个特征列和一个标签列。在这个示例中,我们使用了一个 VectorAssembler 来将三个特征列组合成一个特征向量列,使用 StringIndexer 将标签列转换为数字标签列。我们使用了一个 70/30 的比例将数据集拆分为训练集和测试集,然后建立决策树模型并使用管道进行训练。最后,我们使用 MulticlassClassificationEvaluator 对模型进行评估,并计算测试集上的准确度。
阅读全文
相关推荐
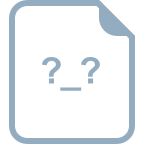
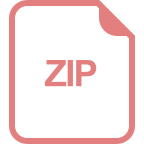
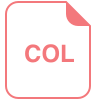
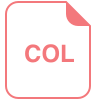
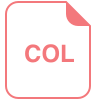
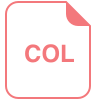
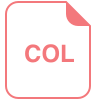
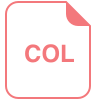
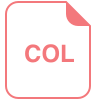
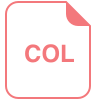
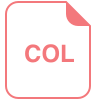
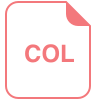
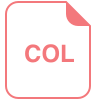
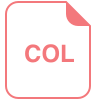
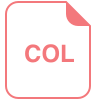
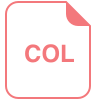