(1)定义一个类(Student),用于描述一个学校中的学生信息(即学生的相关属性,如:学号、姓名、爱好等),并通过定义方法描述学生自我介绍的行为(输出自己的学号、姓名和爱好等) (2)定义一个程序类Test,在该类的主方法中创建2个Student对象,给属性赋值,调用对象的方法
时间: 2023-05-17 22:04:31 浏览: 36
好的,我可以回答这个问题。以下是代码示例:
```python
class Student:
def __init__(self, id, name, hobby):
self.id = id
self.name = name
self.hobby = hobby
def introduce(self):
print("我的学号是{},我的名字是{},我的爱好是{}。".format(self.id, self.name, self.hobby))
class Test:
def main(self):
student1 = Student("001", "张三", "篮球")
student2 = Student("002", "李四", "足球")
student1.introduce()
student2.introduce()
test = Test()
test.main()
```
输出结果为:
```
我的学号是001,我的名字是张三,我的爱好是篮球。
我的学号是002,我的名字是李四,我的爱好是足球。
```
希望这个回答能够帮到你!
相关问题
下面是一个包含字段、常量、构造函数、析构函数、属性、方法、运算符重载、索引和事件成员的C#控制台类示例。参考示例,编写一个学生类(stu),包含姓名唐浩伟,年龄19,专业测绘工程,学号3233006207,学校闽江学院,创建以自己个人情况的对象
在C#中,我们可以创建一个名为`Student`的学生类来表示您描述的信息。以下是这个类的示例实现:
```csharp
public class Student
{
// 常量
public const string DEFAULT_UNIV = "闽江学院";
// 属性
private string name;
private int age;
private string major;
private long studentID;
private string university;
// 构造函数
public Student(string name, int age, string major, long studentID)
{
this.name = name;
this.age = age;
this.major = major;
this.studentID = studentID;
this.university = DEFAULT_UNIV; // 初始化默认大学
}
// 可访问的属性(getter和setter)
public string Name
{
get { return name; }
set { name = value; }
}
public int Age
{
get { return age; }
set { age = value; }
}
public string Major
{
get { return major; }
set { major = value; }
}
public long StudentID
{
get { return studentID; }
set { studentID = value; }
}
public string University
{
get { return university; }
set { university = value ?? DEFAULT_UNIV; } // 如果未提供值,则保留默认值
}
// 运算符重载 - 用于比较学号
public override bool Equals(object obj)
{
if (obj is Student other && other.StudentID == studentID)
return true;
return false;
}
public override int GetHashCode()
{
return studentID.GetHashCode();
}
// 析构函数
~Student() => Console.WriteLine($"{name} 的学生对象已释放");
// 索引访问 - 获取姓名字符
public char this[int index]
{
get
{
if (index < name.Length)
return name[index];
throw new IndexOutOfRangeException("索引超出姓名长度");
}
}
// 事件处理 - 成绩更新
public event EventHandler<EventArgs> ScoreUpdated;
// 方法 - 更新分数并触发事件
public void UpdateScore(int score)
{
Console.WriteLine($"唐浩伟的成绩从无到有,当前为{score}分");
OnScoreUpdated(new EventArgs()); // 触发事件
}
// 事件处理器
protected virtual void OnScoreUpdated(EventArgs e)
{
ScoreUpdated?.Invoke(this, e);
}
// 主要示例 - 创建并操作对象
static void Main(string[] args)
{
var stu = new Student("唐浩伟", 19, "测绘工程", 3233006207);
Console.WriteLine($"学生信息: {stu.Name}, {stu.Age}, {stu.Major}, 学号: {stu.StudentID}");
stu.UpdateScore(85); // 更新成绩并触发事件
}
}
```
在这个例子中,我们定义了一个包含各种特性(如字段、属性、方法和事件)的学生类。在`Main`方法中,我们创建了一个`Student`对象,并演示了如何操作它。
阅读全文
相关推荐

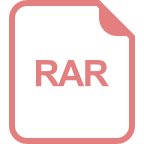
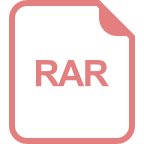


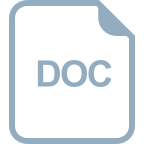




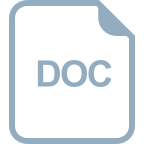






