winform TextBox 重写
时间: 2023-07-29 14:07:56 浏览: 135
若要重写 WinForm 的 TextBox 控件,您可以继承原本的 TextBox 类,并且重写它的方法和属性。以下是一个例子:
```csharp
public class MyTextBox : TextBox
{
public MyTextBox()
{
// 设置一些默认属性
this.Multiline = true;
this.ScrollBars = ScrollBars.Vertical;
}
// 重写 OnPaint 方法,可以自定义绘制样式
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
// 在这里可以添加自定义的绘制逻辑
}
// 重写 OnTextChanged 方法,在文本改变时进行一些额外的操作
protected override void OnTextChanged(EventArgs e)
{
base.OnTextChanged(e);
// 在这里可以添加一些文本改变时的逻辑
}
}
```
在上面的例子中,我们继承了 TextBox 类,并且设置了一些默认属性。我们还重写了 OnPaint 和 OnTextChanged 方法,以便在文本框绘制和文本改变时进行自定义的操作。您可以根据您的需求添加更多的重写方法和属性。
相关问题
winform TextBox 透明
要在 WinForm 中使 TextBox 控件透明,您需要执行以下步骤:
1. 将 TextBox 控件的 BackColor 设置为 Transparent(即透明)。
```csharp
textBox1.BackColor = Color.Transparent;
```
2. 将 TextBox 控件的 Parent 控件(例如 Form、Panel 等)的 DoubleBuffered 属性设置为 true,以避免闪烁问题。
```csharp
this.DoubleBuffered = true;
```
3. 重写 TextBox 控件的 OnPaintBackground 方法,并在其中绘制父控件的背景。
```csharp
protected override void OnPaintBackground(PaintEventArgs e)
{
base.OnPaintBackground(e);
if (Parent != null)
{
// 计算 TextBox 相对于父控件的位置
Rectangle rect = new Rectangle(this.Left, this.Top, this.Width, this.Height);
e.Graphics.TranslateTransform(Parent.Left - Left, Parent.Top - Top);
try
{
// 绘制父控件的背景
Parent.DrawToBitmap(e.Graphics, rect);
}
finally
{
e.Graphics.TranslateTransform(Left - Parent.Left, Top - Parent.Top);
}
}
}
```
完整的代码如下:
```csharp
public class MyTextBox : TextBox
{
public MyTextBox()
{
this.BackColor = Color.Transparent;
}
protected override void OnPaintBackground(PaintEventArgs e)
{
base.OnPaintBackground(e);
if (Parent != null)
{
// 计算 TextBox 相对于父控件的位置
Rectangle rect = new Rectangle(this.Left, this.Top, this.Width, this.Height);
e.Graphics.TranslateTransform(Parent.Left - Left, Parent.Top - Top);
try
{
// 绘制父控件的背景
Parent.DrawToBitmap(e.Graphics, rect);
}
finally
{
e.Graphics.TranslateTransform(Left - Parent.Left, Top - Parent.Top);
}
}
}
}
```
使用这个自定义的 MyTextBox 控件时,您可以将其放在一个透明的 Panel 控件中,或者将其设置为窗体的背景,从而实现 TextBox 控件的透明效果。
winform textbox 上下拉杆
Winform的TextBox控件是一个文本输入框,在使用过程中可以通过设置属性来实现上下拉杆的效果。具体的方法有两种:
1. 将TextBox设置为多行模式(Multiline=True),然后设置滚动条(ScrollBars=Vertical)。这样就可以在TextBox中显示垂直滚动条,实现上下拉杆的效果。
2. 继承系统的TextBox控件,并重写相应的方法,实现垂直居中显示和自动折行(WordWrap=True)。通过这种方式,可以在TextBox中实现垂直居中的文本,并自动换行。
阅读全文
相关推荐
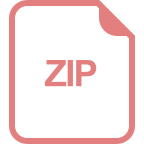













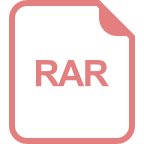