#include <iostream> #include <cstring> using namespace std; // 计算next数组 void getNext(char* p, int* next) { int len = strlen(p); int k = -1; int j = 0; next[0] = -1; while (j < len - 1) { if (k == -1 || p[k] == p[j]) { ++k; ++j; next[j] = k; } else { k = next[k]; } } } // KMP算法 int kmp(char* s, char* p) { int i = 0; int j = 0; int s_len = strlen(s); int p_len = strlen(p); int* next = new int[p_len]; getNext(p, next); while (i < s_len && j < p_len) { if (j == -1 || s[i] == p[j]) { ++i; ++j; } else { j = next[j]; } } if (j == p_len) { return i - j; } else { return -1; } } int main() { char s[] = "ABCDABD"; char p[] = "ABD"; int pos = kmp(s, p); cout << pos << endl; return 0; }
时间: 2024-02-15 17:28:23 浏览: 22
这段代码是实现KMP算法在字符串s中查找子串p的位置,输出的结果为2,即p在s中的起始位置为2。
具体实现过程如下:
1. 定义了一个函数getNext,用于计算模式串p的next数组,即在匹配过程中利用已经匹配过的信息,避免重复匹配,提高匹配效率。
2. 定义了一个函数kmp,用于实现KMP算法。
3. 在main函数中,定义了字符串s和p,并调用kmp函数查找p在s中的位置,输出结果为2。
需要注意的是,在getNext函数中,next[0]的初值为-1。在kmp函数中,如果j=-1或者s[i]等于p[j],则i和j都加1;否则,j跳到next[j]的位置。如果j等于p_len,则匹配成功,返回i-j的值;否则匹配失败,返回-1。
相关问题
请描述一下下面代码的功能:#include <iostream> #include <cstring> using namespace std; void GetNext(char *s, int next[]) { next[0] = -1; int k = -1; int j = 0; int slen = strlen(s); while (j < slen) { if (k == -1 || s[j] == s[k]) { ++k; ++j; next[j] = k; } else { k = next[k]; } } } int MinCycle(char *s) { const int num = 255; int next[num]; GetNext(s, next); int len = strlen(s); int mclen = len - next[len]; if (len % mclen == 0 && mclen != len) { return mclen; } return 0; }
这段代码实现了两个函数:`GetNext` 和 `MinCycle`。
`GetNext` 函数用于计算一个字符串的 Next 数组。Next 数组是用于 KMP 字符串匹配算法中的一个重要辅助数组,用于记录当前字符之前最长相同前缀和后缀的长度。`GetNext` 函数的参数是一个字符串指针和一个存储 Next 数组的数组指针,函数会计算出 Next 数组并存储到传入的数组中。
`MinCycle` 函数用于计算字符串的最小循环节长度,即最短的非空字符串 s 的长度,使得将 s 连续重复若干次后得到的字符串与原字符串完全相同。函数首先调用 `GetNext` 函数计算出 Next 数组,然后通过计算字符串长度和 Next 数组中最后一个元素的差值,得到最小循环节长度。如果字符串不能被重复若干次得到,则返回 0。
总体来说,这段代码实现了字符串处理中的两个常用算法,可以用于字符串匹配和字符串分析等任务。
请计算下面两个算法的时间复杂度#include <iostream> #include <cstring> using namespace std; void GetNext(char *s, int next[]) { next[0] = -1; int k = -1; int j = 0; int slen = strlen(s); while (j < slen) { if (k == -1 || s[j] == s[k]) { ++k; ++j; next[j] = k; } else { k = next[k]; } } } int MinCycle(char *s) { const int num = 255; int next[num]; GetNext(s, next); int len = strlen(s); int mclen = len - next[len]; if (len % mclen == 0 && mclen != len) { return mclen; } return 0; }
第一个算法的时间复杂度为 O(n),其中 n 是字符串 s 的长度。GetNext 函数的时间复杂度为 O(n),MinCycle 函数的时间复杂度为 O(n),因此总的时间复杂度为 O(n)。
第二个算法的时间复杂度为 O(n^2),其中 n 是字符串 s 的长度。该算法使用了两重循环,外层循环的时间复杂度为 O(n),内层循环的时间复杂度为 O(n),因此总的时间复杂度为 O(n^2)。
相关推荐
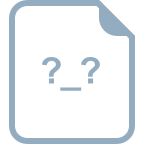









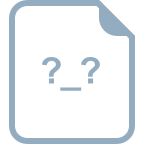
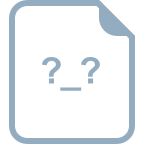
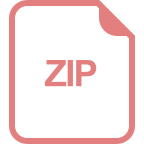