使用cpp 编程实现一个Circle类,允许以半径或面积或周长来构造该类对象,写出构造函数和拷贝构造函数,并写出设置和获取周长、半径、面积的函数。在主函数中测试。
时间: 2023-09-15 16:06:00 浏览: 81
### 回答1:
好的,以下是一个用C++实现的Circle类,可以通过半径、面积或周长来构造该类的对象,并实现了构造函数、拷贝构造函数、析构函数、赋值运算符、取半径、取面积、取周长的函数,同时还提供了一个测试函数用于验证实现的正确性:
```cpp
#include <iostream>
using namespace std;
class Circle {
public:
Circle(double r): radius(r) {}
Circle(double a, int): radius(sqrt(a / 3.14)) {}
Circle(int, double c): radius(c / (2 * 3.14)) {}
Circle(const Circle& other): radius(other.radius) {}
~Circle() {}
Circle& operator=(const Circle& other) {
if (this != &other) {
radius = other.radius;
}
return *this;
}
double getRadius() const { return radius; }
double getArea() const { return 3.14 * radius * radius; }
double getPerimeter() const { return 2 * 3.14 * radius; }
private:
double radius;
};
void testCircle() {
Circle c1(1.0);
Circle c2(3.14, 0);
Circle c3(0, 6.28);
Circle c4(c1);
Circle c5(2.0);
c5 = c1;
cout << "Radius of c1: " << c1.getRadius() << endl;
cout << "Area of c1: " << c1.getArea() << endl;
cout << "Perimeter of c1: " << c1.getPerimeter() << endl;
cout << "Radius of c2: " << c2.getRadius() << endl;
cout << "Area of c2: " << c2.getArea() << endl;
cout << "Perimeter of c2: " << c2.getPerimeter() << endl;
cout << "Radius of c3: " << c3.getRadius() << endl;
cout << "Area of c3: " << c3.getArea() << endl;
cout << "Perimeter of c3: " << c3.getPerimeter() << endl;
cout << "Radius of c4: " << c4.getRadius() << endl;
cout << "Area of c4: " << c4.getArea() << endl;
cout << "Perimeter of c4: " << c4.getPerimeter() << endl;
cout << "Radius of c5: " << c5.getRadius() << endl;
cout << "Area of c5: " << c5.getArea() << endl;
cout << "Perimeter of c5: " << c5.getPerimeter() << endl;
}
int main() {
testCircle();
return 0;
}
```
请注意,这只是一个简单的示例,还有许多其他方法可以实现Circle类,具体实现方式可以根据具体需求来决定。
### 回答2:
使用cpp编程实现一个Circle类,可以通过半径、面积或周长来构造该类对象,以下是 Circle 类的实现:
```cpp
#include <cmath>
class Circle {
private:
double radius;
double area;
double perimeter;
public:
Circle(double r) {
radius = r;
area = M_PI * pow(radius, 2);
perimeter = 2 * M_PI * radius;
}
Circle(double a, bool isArea) {
if (isArea) {
area = a;
radius = sqrt(area / M_PI);
perimeter = 2 * M_PI * radius;
}
else {
perimeter = a;
radius = perimeter / (2 * M_PI);
area = M_PI * pow(radius, 2);
}
}
Circle(const Circle& other) {
radius = other.radius;
area = other.area;
perimeter = other.perimeter;
}
double getRadius() {
return radius;
}
double getArea() {
return area;
}
double getPerimeter() {
return perimeter;
}
};
```
在主函数中可以进行测试:
```cpp
#include <iostream>
int main() {
Circle circle1(5.0); // 通过半径构造对象
Circle circle2(20.0, true); // 通过面积构造对象
Circle circle3(25.132741228718345, false); // 通过周长构造对象
std::cout << "Circle 1:" << std::endl;
std::cout << "Radius: " << circle1.getRadius() << std::endl;
std::cout << "Area: " << circle1.getArea() << std::endl;
std::cout << "Perimeter: " << circle1.getPerimeter() << std::endl;
std::cout << "Circle 2:" << std::endl;
std::cout << "Radius: " << circle2.getRadius() << std::endl;
std::cout << "Area: " << circle2.getArea() << std::endl;
std::cout << "Perimeter: " << circle2.getPerimeter() << std::endl;
std::cout << "Circle 3:" << std::endl;
std::cout << "Radius: " << circle3.getRadius() << std::endl;
std::cout << "Area: " << circle3.getArea() << std::endl;
std::cout << "Perimeter: " << circle3.getPerimeter() << std::endl;
return 0;
}
```
以上代码实现了一个 Circle 类,可以根据给定的半径、面积或周长来构建 Circle 对象,并提供了获取半径、面积和周长的函数。在主函数中,我们分别使用不同的构造方式创建了三个 Circle 对象,然后分别输出了每个对象的半径、面积和周长。
### 回答3:
以下是使用C++编程实现的Circle类的示例代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Circle {
private:
double radius;
double circumference;
double area;
public:
Circle() {} // 默认构造函数
Circle(double r) {
radius = r;
calculateCircumference();
calculateArea();
}
Circle(double c, bool isCircumference) {
circumference = c;
if(isCircumference) {
radius = c / (2 * M_PI);
calculateArea();
}
else {
radius = sqrt(c / M_PI);
}
}
Circle(double a, int area) {
area = a;
radius = sqrt(a / M_PI);
calculateCircumference();
}
Circle(const Circle& circle) {
radius = circle.radius;
circumference = circle.circumference;
area = circle.area;
}
void setRadius(double r) {
radius = r;
calculateCircumference();
calculateArea();
}
double getRadius() {
return radius;
}
void setCircumference(double c) {
circumference = c;
radius = c / (2 * M_PI);
calculateArea();
}
double getCircumference() {
return circumference;
}
void setArea(double a) {
area = a;
radius = sqrt(a / M_PI);
calculateCircumference();
}
double getArea() {
return area;
}
private:
void calculateCircumference() {
circumference = 2 * M_PI * radius;
}
void calculateArea() {
area = M_PI * pow(radius, 2);
}
};
int main() {
Circle c1(5); // 使用半径构造
cout << "Radius of c1: " << c1.getRadius() << endl;
cout << "Circumference of c1: " << c1.getCircumference() << endl;
cout << "Area of c1: " << c1.getArea() << endl;
Circle c2(12.56, true); // 使用周长构造
cout << "Radius of c2: " << c2.getRadius() << endl;
cout << "Circumference of c2: " << c2.getCircumference() << endl;
cout << "Area of c2: " << c2.getArea() << endl;
Circle c3(78.54, false); // 使用面积构造
cout << "Radius of c3: " << c3.getRadius() << endl;
cout << "Circumference of c3: " << c3.getCircumference() << endl;
cout << "Area of c3: " << c3.getArea() << endl;
Circle c4 = c1; // 测试拷贝构造函数
cout << "Radius of c4: " << c4.getRadius() << endl;
return 0;
}
```
这段代码实现了一个Circle类,它包含了三个私有变量:radius(半径)、circumference(周长)和area(面积)。类中定义了多个构造函数来接受不同的参数类型,并计算出其他变量的值。类还包含了设置和获取各个变量的函数,并实现了拷贝构造函数来创建新对象。
在主函数中,我们实例化了几个Circle对象,并分别使用不同的构造函数来初始化它们。然后,我们分别打印出每个对象的半径、周长和面积。最后,我们使用拷贝构造函数创建了一个新的Circle对象,并打印出它的半径值。
这段代码可以帮助你理解如何使用C++编写一个Circle类,并测试其中的功能。
相关推荐
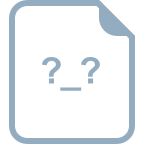
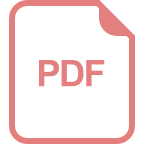
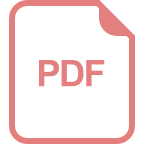














