用C语言做一个学生系统 功能1.添加学生信息2.删除学生信息3.修改学生信息4.遍历学生信息0.退出系统 并且添加学生信息要输入学号姓名 成绩并且用中文输出
时间: 2023-08-22 07:05:05 浏览: 43
以下是一个简单的C语言学生系统程序,实现了添加、删除、修改和遍历学生信息的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 100 // 最大学生数
struct student {
int id; // 学号
char name[20]; // 姓名
float score; // 成绩
};
struct student students[MAX_STUDENT_NUM]; // 学生数组
int student_num = 0; // 学生数目
int add_student() {
if (student_num >= MAX_STUDENT_NUM) {
printf("学生数目已达到最大值!\n");
return 0;
}
struct student s;
printf("请输入学号:");
scanf("%d", &(s.id));
printf("请输入姓名:");
scanf("%s", s.name);
printf("请输入成绩:");
scanf("%f", &(s.score));
students[student_num++] = s;
printf("添加成功!\n");
return 1;
}
int delete_student() {
int id, i, j;
printf("请输入要删除的学生学号:");
scanf("%d", &id);
for (i = 0; i < student_num; i++) {
if (students[i].id == id) {
for (j = i; j < student_num - 1; j++) {
students[j] = students[j + 1];
}
student_num--;
printf("删除成功!\n");
return 1;
}
}
printf("找不到学号为 %d 的学生!\n", id);
return 0;
}
int modify_student() {
int id, i;
printf("请输入要修改的学生学号:");
scanf("%d", &id);
for (i = 0; i < student_num; i++) {
if (students[i].id == id) {
printf("请输入新的姓名(留空表示不修改):");
scanf("%s", students[i].name);
printf("请输入新的成绩(留空表示不修改):");
scanf("%f", &(students[i].score));
printf("修改成功!\n");
return 1;
}
}
printf("找不到学号为 %d 的学生!\n", id);
return 0;
}
void traverse_student() {
int i;
printf("学号\t姓名\t成绩\n");
for (i = 0; i < student_num; i++) {
printf("%d\t%s\t%.2f\n", students[i].id, students[i].name, students[i].score);
}
}
int main() {
int choice;
while (1) {
printf("\n请选择操作:\n");
printf("1. 添加学生信息\n");
printf("2. 删除学生信息\n");
printf("3. 修改学生信息\n");
printf("4. 遍历学生信息\n");
printf("0. 退出系统\n");
printf("请输入操作编号:");
scanf("%d", &choice);
switch (choice) {
case 0:
printf("谢谢使用!\n");
exit(0);
case 1:
add_student();
break;
case 2:
delete_student();
break;
case 3:
modify_student();
break;
case 4:
traverse_student();
break;
default:
printf("无效的操作编号!\n");
break;
}
}
return 0;
}
```
注意,这个程序只是一个简单的示例,没有进行错误处理和数据持久化等操作。在实际应用中还需要根据具体需求进行进一步开发。
相关推荐
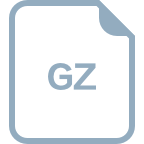
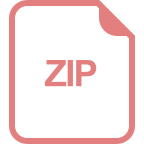
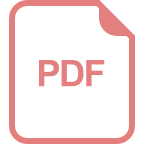














