用C语言实现酒水管理系统。
时间: 2023-05-16 17:06:53 浏览: 113
非常感谢您的提问。以下是一个简单的酒水管理系统的C语言实现:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DRINKS 100
typedef struct {
char name[50];
float price;
int quantity;
} Drink;
Drink drinks[MAX_DRINKS];
int num_drinks = 0;
void add_drink() {
if (num_drinks == MAX_DRINKS) {
printf("Sorry, the maximum number of drinks has been reached.\n");
return;
}
Drink new_drink;
printf("Enter the name of the drink: ");
scanf("%s", new_drink.name);
printf("Enter the price of the drink: ");
scanf("%f", &new_drink.price);
printf("Enter the quantity of the drink: ");
scanf("%d", &new_drink.quantity);
drinks[num_drinks] = new_drink;
num_drinks++;
printf("Drink added successfully.\n");
}
void list_drinks() {
printf("Name\tPrice\tQuantity\n");
for (int i = 0; i < num_drinks; i++) {
printf("%s\t%.2f\t%d\n", drinks[i].name, drinks[i].price, drinks[i].quantity);
}
}
void sell_drink() {
char drink_name[50];
int quantity;
printf("Enter the name of the drink you want to sell: ");
scanf("%s", drink_name);
printf("Enter the quantity you want to sell: ");
scanf("%d", &quantity);
for (int i = 0; i < num_drinks; i++) {
if (strcmp(drinks[i].name, drink_name) == 0) {
if (drinks[i].quantity < quantity) {
printf("Sorry, there are not enough %s in stock.\n", drink_name);
return;
}
drinks[i].quantity -= quantity;
printf("Sold %d %s for a total of %.2f.\n", quantity, drink_name, quantity * drinks[i].price);
return;
}
}
printf("Sorry, we do not have %s in stock.\n", drink_name);
}
int main() {
int choice;
do {
printf("1. Add a drink\n");
printf("2. List all drinks\n");
printf("3. Sell a drink\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_drink();
break;
case 2:
list_drinks();
break;
case 3:
sell_drink();
break;
case 4:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while (choice != 4);
return 0;
}
阅读全文
相关推荐
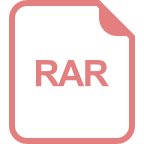
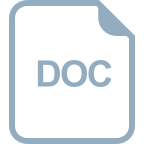





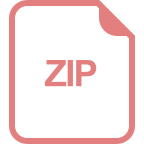
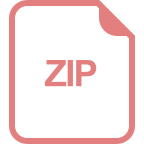
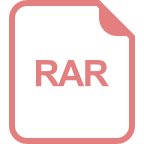
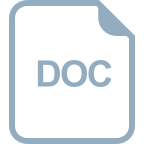
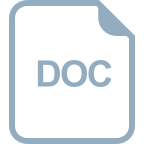
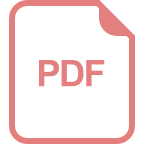