Android中用opencv得到一个图像光斑的中心
时间: 2023-11-13 11:02:26 浏览: 85
在Android平台中,也可以使用OpenCV来得到一个图像光斑的中心。具体步骤与PC端类似,主要分为二值化和轮廓提取、计算轮廓重心两个步骤。以下是一个示例代码,可以找出图像中所有光斑的中心:
```java
import org.opencv.android.Utils;
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.core.MatOfPoint;
import org.opencv.core.MatOfPoint2f;
import org.opencv.core.Point;
import org.opencv.imgproc.Imgproc;
import java.util.ArrayList;
import java.util.List;
public class SpotDetector {
public static List<Point> detect(Mat image) {
// 转换为灰度图像
Mat gray = new Mat();
Imgproc.cvtColor(image, gray, Imgproc.COLOR_BGR2GRAY);
// 二值化处理
Mat binary = new Mat();
Imgproc.threshold(gray, binary, 0, 255, Imgproc.THRESH_BINARY | Imgproc.THRESH_OTSU);
// 找出轮廓
List<MatOfPoint> contours = new ArrayList<>();
Mat hierarchy = new Mat();
Imgproc.findContours(binary, contours, hierarchy, Imgproc.RETR_EXTERNAL, Imgproc.CHAIN_APPROX_SIMPLE);
// 计算轮廓重心并输出
List<Point> centers = new ArrayList<>();
for (int i = 0; i < contours.size(); i++) {
// 计算轮廓重心
MatOfPoint2f contour2f = new MatOfPoint2f(contours.get(i).toArray());
Moments moments = Imgproc.moments(contour2f);
Point center = new Point(moments.m10 / moments.m00, moments.m01 / moments.m00);
// 输出重心坐标
System.out.println("Center of spot " + i + " is (" + center.x + ", " + center.y + ")");
centers.add(center);
}
return centers;
}
}
```
在上面的代码中,我们首先将输入图像转换为灰度图像,然后进行二值化处理,接着使用cv::findContours函数找出轮廓,并使用cv::moments函数计算轮廓重心。由于Java没有C++中的cv::Point2f类型,因此我们使用了cv::Point类型来表示重心坐标。
在Android平台上使用OpenCV需要在项目中导入OpenCV库,具体可以参考OpenCV官方文档。
阅读全文
相关推荐
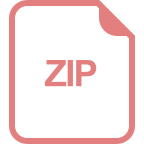
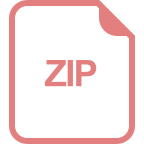
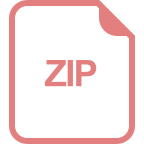
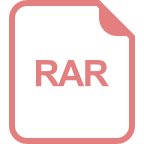
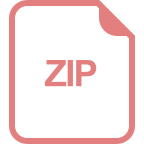
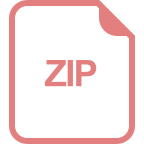
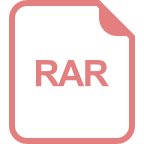
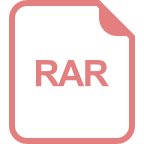
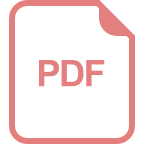
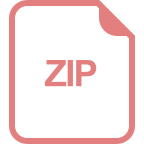
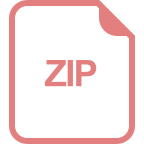
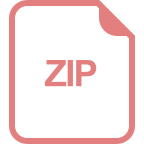
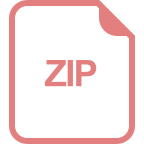
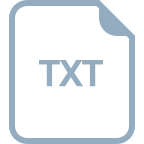