使用pytorch编写基于粒子群优化的回声状态网络,用于分类
时间: 2023-05-17 22:07:31 浏览: 78
非常感谢您的问题。基于粒子群优化的回声状态网络是一种有效的分类算法,可以使用PyTorch进行实现。以下是一个基于PyTorch的粒子群优化回声状态网络的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
class PSOESN(nn.Module):
def __init__(self, input_size, hidden_size, output_size, spectral_radius=0.9, p=0.5, c1=2.0, c2=2.0, w=0.7):
super(PSOESN, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.spectral_radius = spectral_radius
self.p = p
self.c1 = c1
self.c2 = c2
self.w = w
self.W_in = nn.Parameter(torch.randn(hidden_size, input_size))
self.W = nn.Parameter(torch.randn(hidden_size, hidden_size))
self.W_out = nn.Parameter(torch.randn(output_size, hidden_size))
self.bias = nn.Parameter(torch.randn(hidden_size))
self.activation = nn.Tanh()
def forward(self, input):
x = torch.zeros(self.hidden_size)
for i in range(input.shape[0]):
u = input[i]
x = (1 - self.p) * x + self.p * self.activation(torch.matmul(self.W_in, u) + torch.matmul(self.W, x) + self.bias)
y = torch.matmul(self.W_out, x)
return y
def fit(self, X, y, swarm_size=10, max_iter=100):
X = torch.tensor(X, dtype=torch.float32)
y = torch.tensor(y, dtype=torch.float32)
n = X.shape[0]
best_particle = None
best_fitness = float('inf')
particles = []
for i in range(swarm_size):
particle = {'W_in': torch.randn_like(self.W_in),
'W': torch.randn_like(self.W),
'W_out': torch.randn_like(self.W_out),
'bias': torch.randn_like(self.bias)}
particles.append(particle)
for i in range(max_iter):
for particle in particles:
model = PSOESN(self.input_size, self.hidden_size, self.output_size, self.spectral_radius, self.p)
model.W_in = nn.Parameter(particle['W_in'])
model.W = nn.Parameter(particle['W'])
model.W_out = nn.Parameter(particle['W_out'])
model.bias = nn.Parameter(particle['bias'])
y_pred = model(X)
loss = nn.MSELoss()(y_pred, y)
fitness = loss.item()
if fitness < best_fitness:
best_fitness = fitness
best_particle = particle
v_W_in = torch.randn_like(self.W_in)
v_W = torch.randn_like(self.W)
v_W_out = torch.randn_like(self.W_out)
v_bias = torch.randn_like(self.bias)
v_W_in = self.w * v_W_in + self.c1 * torch.rand_like(v_W_in) * (best_particle['W_in'] - particle['W_in']) + self.c2 * torch.rand_like(v_W_in) * (self.W_in - particle['W_in'])
v_W = self.w * v_W + self.c1 * torch.rand_like(v_W) * (best_particle['W'] - particle['W']) + self.c2 * torch.rand_like(v_W) * (self.W - particle['W'])
v_W_out = self.w * v_W_out + self.c1 * torch.rand_like(v_W_out) * (best_particle['W_out'] - particle['W_out']) + self.c2 * torch.rand_like(v_W_out) * (self.W_out - particle['W_out'])
v_bias = self.w * v_bias + self.c1 * torch.rand_like(v_bias) * (best_particle['bias'] - particle['bias']) + self.c2 * torch.rand_like(v_bias) * (self.bias - particle['bias'])
particle['W_in'] += v_W_in
particle['W'] += v_W
particle['W_out'] += v_W_out
particle['bias'] += v_bias
self.W_in = nn.Parameter(best_particle['W_in'])
self.W = nn.Parameter(best_particle['W'])
self.W_out = nn.Parameter(best_particle['W_out'])
self.bias = nn.Parameter(best_particle['bias'])
# Example usage
input_size = 10
hidden_size = 100
output_size = 1
X = np.random.randn(1000, input_size)
y = np.random.randn(1000, output_size)
model = PSOESN(input_size, hidden_size, output_size)
model.fit(X, y)
y_pred = model(torch.tensor(X, dtype=torch.float32))
print(y_pred)
```
希望这个代码示例能够帮助您实现基于粒子群优化的回声状态网络。
阅读全文
相关推荐
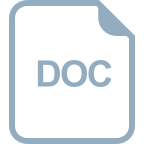
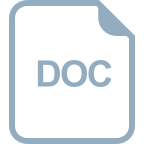
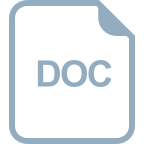
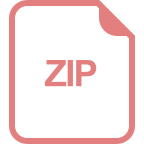
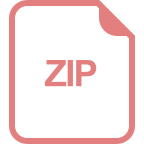
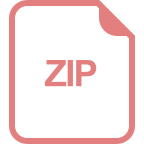
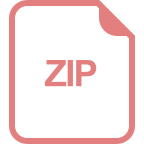
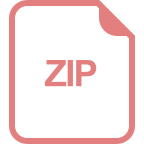
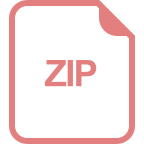
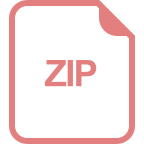
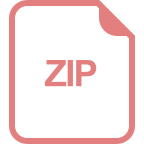
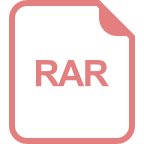
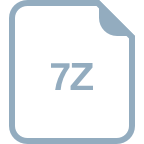
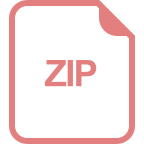
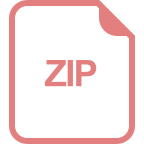
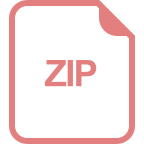
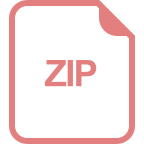