利用matlab提供的低通滤波器实现图像信号的滤波运算,包括理想低通滤波器、巴特沃
时间: 2023-11-26 13:06:02 浏览: 36
思低通滤波器和高斯低通滤波器两种。
1. 理想低通滤波器
理想低通滤波器可以通过以下步骤实现:
1)定义一个矩形函数,表示滤波器的频率响应,其值为1表示通过,0表示阻止,如下所示:
```matlab
function H = ideal_lowpass_filter(D0, M, N)
% 构造理想低通滤波器
% D0: 截止频率
% M, N: 图像大小
% H: 理想低通滤波器
u = 0:(M-1);
v = 0:(N-1);
idx = find(u > M/2);
u(idx) = u(idx) - M;
idy = find(v > N/2);
v(idy) = v(idy) - N;
[V, U] = meshgrid(v, u);
D = sqrt(U.^2 + V.^2);
H = double(D <= D0);
end
```
2)将滤波器的频率响应和图像的频率域表示进行卷积,得到滤波后的图像,如下所示:
```matlab
function img_filtered = ideal_lowpass_filtering(img, D0)
% 理想低通滤波器滤波
% img: 待滤波图像
% D0: 截止频率
% img_filtered: 滤波后的图像
[M, N] = size(img);
H = ideal_lowpass_filter(D0, M, N);
F = fft2(double(img));
F_filtered = H .* F;
img_filtered = real(ifft2(F_filtered));
img_filtered = img_filtered(1:M, 1:N);
end
```
2. 巴特沃思低通滤波器
巴特沃思低通滤波器可以通过以下步骤实现:
1)定义一个巴特沃斯函数,表示滤波器的频率响应,如下所示:
```matlab
function H = butterworth_lowpass_filter(D0, M, N, n)
% 构造巴特沃斯低通滤波器
% D0: 截止频率
% M, N: 图像大小
% n: 巴特沃斯滤波器阶数
% H: 巴特沃斯低通滤波器
u = 0:(M-1);
v = 0:(N-1);
idx = find(u > M/2);
u(idx) = u(idx) - M;
idy = find(v > N/2);
v(idy) = v(idy) - N;
[V, U] = meshgrid(v, u);
D = sqrt(U.^2 + V.^2);
H = 1 ./ (1 + (D./D0).^(2*n));
end
```
2)将滤波器的频率响应和图像的频率域表示进行卷积,得到滤波后的图像,如下所示:
```matlab
function img_filtered = butterworth_lowpass_filtering(img, D0, n)
% 巴特沃斯低通滤波器滤波
% img: 待滤波图像
% D0: 截止频率
% n: 巴特沃斯滤波器阶数
% img_filtered: 滤波后的图像
[M, N] = size(img);
H = butterworth_lowpass_filter(D0, M, N, n);
F = fft2(double(img));
F_filtered = H .* F;
img_filtered = real(ifft2(F_filtered));
img_filtered = img_filtered(1:M, 1:N);
end
```
3. 高斯低通滤波器
高斯低通滤波器可以通过以下步骤实现:
1)定义一个高斯函数,表示滤波器的频率响应,如下所示:
```matlab
function H = gaussian_lowpass_filter(D0, M, N)
% 构造高斯低通滤波器
% D0: 截止频率
% M, N: 图像大小
% H: 高斯低通滤波器
u = 0:(M-1);
v = 0:(N-1);
idx = find(u > M/2);
u(idx) = u(idx) - M;
idy = find(v > N/2);
v(idy) = v(idy) - N;
[V, U] = meshgrid(v, u);
D = sqrt(U.^2 + V.^2);
H = exp(-(D.^2)./(2*(D0.^2)));
end
```
2)将滤波器的频率响应和图像的频率域表示进行卷积,得到滤波后的图像,如下所示:
```matlab
function img_filtered = gaussian_lowpass_filtering(img, D0)
% 高斯低通滤波器滤波
% img: 待滤波图像
% D0: 截止频率
% img_filtered: 滤波后的图像
[M, N] = size(img);
H = gaussian_lowpass_filter(D0, M, N);
F = fft2(double(img));
F_filtered = H .* F;
img_filtered = real(ifft2(F_filtered));
img_filtered = img_filtered(1:M, 1:N);
end
```
相关推荐
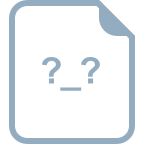
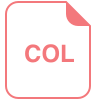
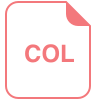
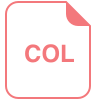
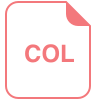
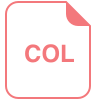









