python 实时访问服务器文件
时间: 2023-11-25 08:14:03 浏览: 31
要实现 Python 实时访问服务器文件,可以使用 paramiko 库连接远程服务器,并使用 SFTP 协议实时获取文件内容。以下是一个示例代码:
```python
import paramiko
import time
# 远程服务器信息
hostname = 'your_remote_host'
port = 22
username = 'your_username'
password = 'your_password'
# 连接远程服务器
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(hostname=hostname, port=port, username=username, password=password)
# 建立 SFTP 通道
sftp = ssh.open_sftp()
# 实时获取文件内容
remote_file = '/path/to/remote/file'
local_file = '/path/to/local/file'
while True:
try:
# 获取文件内容
with sftp.open(remote_file) as remote_f, open(local_file, 'w') as local_f:
for line in remote_f:
local_f.write(line)
print(line.strip())
except KeyboardInterrupt:
# 用户中断程序
print('User interrupt')
break
except:
# 重新连接服务器
print('Connection lost, try to reconnect...')
ssh.connect(hostname=hostname, port=port, username=username, password=password)
sftp = ssh.open_sftp()
time.sleep(1)
# 关闭 SFTP 通道和 SSH 连接
sftp.close()
ssh.close()
```
在上述代码中,通过循环实时获取远程文件内容。当用户按下 Ctrl+C 中断程序时,程序退出循环并关闭连接。如果连接中断,程序会自动重新连接。需要将 `your_remote_host`、`your_username` 和 `your_password` 替换为实际的远程服务器信息。`/path/to/remote/file` 表示远程服务器上的文件路径,`/path/to/local/file` 表示本地文件路径。利用 `sftp.open` 方法可以打开远程文件,并通过 `for` 循环逐行读取文件内容。在每次读取文件内容时,将内容写入本地文件并打印出来。
相关推荐
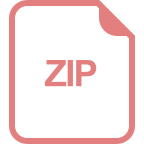
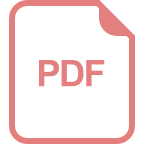
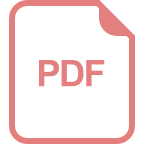














