django 缓存使用场景及示例
时间: 2023-08-21 19:01:53 浏览: 53
Django 缓存可以用于存储一些计算结果、数据库查询结果、模板渲染结果等,从而避免重复计算、查询数据库等操作,提高应用性能。下面是一些 Django 缓存的使用场景及示例:
1. 存储计算结果
如果应用中有一些计算操作比较耗时,可以将计算结果存储到缓存中,下次需要使用时直接从缓存中获取,避免重复计算。例如:
``` python
from django.core.cache import cache
def get_fibonacci_num(n):
if n < 2:
return n
else:
key = 'fibonacci_{}'.format(n)
result = cache.get(key)
if result is not None:
return result
else:
result = get_fibonacci_num(n-1) + get_fibonacci_num(n-2)
cache.set(key, result)
return result
```
以上代码使用缓存存储了斐波那契数列的计算结果,避免了重复计算。
2. 存储数据库查询结果
如果应用中有一些查询操作比较耗时,可以将查询结果存储到缓存中,下次需要使用时直接从缓存中获取,避免重复查询数据库。例如:
``` python
from django.core.cache import cache
from myapp.models import Post
def get_latest_posts():
key = 'latest_posts'
result = cache.get(key)
if result is not None:
return result
else:
result = Post.objects.order_by('-pub_date')[:10]
cache.set(key, result)
return result
```
以上代码使用缓存存储了最新的 10 篇文章的查询结果,避免了重复查询数据库。
3. 存储模板渲染结果
如果应用中有一些模板渲染操作比较耗时,可以将模板渲染结果存储到缓存中,下次需要使用时直接从缓存中获取,避免重复渲染。例如:
``` python
from django.core.cache import cache
from django.template.loader import render_to_string
def render_latest_posts():
key = 'latest_posts_html'
result = cache.get(key)
if result is not None:
return result
else:
posts = get_latest_posts()
result = render_to_string('latest_posts.html', {'posts': posts})
cache.set(key, result)
return result
```
以上代码使用缓存存储了最新文章的 HTML 渲染结果,避免了重复渲染。
注意:使用缓存可以提高应用性能,但是需要注意缓存的更新和失效机制,避免使用过期的缓存数据。同时,也需要根据实际情况进行选择和优化,避免缓存占用过多的内存等问题。
相关推荐
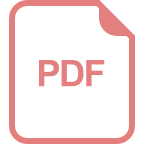
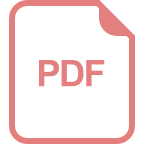














