用C语言写一个有头单向链表存放员工(name age gender),可以实现外部增删查改。
时间: 2023-06-19 16:10:57 浏览: 297
以下是使用C语言实现有头单向链表存放员工信息的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工结构体
typedef struct Employee {
char name[20];
int age;
char gender[10];
struct Employee *next;
} Employee;
// 全局变量,指向头结点
Employee *head = NULL;
// 函数声明
void addEmployee();
void deleteEmployee();
void modifyEmployee();
void searchEmployee();
void printAllEmployees();
int main() {
int choice;
do {
printf("\n1. Add employee\n");
printf("2. Delete employee\n");
printf("3. Modify employee\n");
printf("4. Search employee\n");
printf("5. Print all employees\n");
printf("6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addEmployee();
break;
case 2:
deleteEmployee();
break;
case 3:
modifyEmployee();
break;
case 4:
searchEmployee();
break;
case 5:
printAllEmployees();
break;
case 6:
printf("Exiting program...\n");
break;
default:
printf("Invalid choice. Try again.\n");
}
} while (choice != 6);
return 0;
}
void addEmployee() {
Employee *newEmployee = (Employee *) malloc(sizeof(Employee));
printf("Enter employee name: ");
scanf("%s", newEmployee->name);
printf("Enter employee age: ");
scanf("%d", &newEmployee->age);
printf("Enter employee gender: ");
scanf("%s", newEmployee->gender);
newEmployee->next = NULL;
if (head == NULL) { // 链表为空,新节点为第一个节点
head = newEmployee;
} else { // 链表不为空,将新节点插入到链表头部
newEmployee->next = head;
head = newEmployee;
}
printf("Employee added successfully.\n");
}
void deleteEmployee() {
if (head == NULL) { // 链表为空
printf("No employees to delete.\n");
return;
}
char name[20];
printf("Enter the name of the employee to delete: ");
scanf("%s", name);
Employee *currentEmployee = head;
Employee *previousEmployee = NULL;
int found = 0;
while (currentEmployee != NULL) {
if (strcmp(currentEmployee->name, name) == 0) { // 找到要删除的节点
found = 1;
break;
}
previousEmployee = currentEmployee;
currentEmployee = currentEmployee->next;
}
if (!found) { // 没有找到要删除的节点
printf("Employee not found.\n");
} else {
if (previousEmployee != NULL) { // 要删除的节点不是头结点
previousEmployee->next = currentEmployee->next;
} else { // 要删除的节点是头结点
head = currentEmployee->next;
}
free(currentEmployee);
printf("Employee deleted successfully.\n");
}
}
void modifyEmployee() {
if (head == NULL) { // 链表为空
printf("No employees to modify.\n");
return;
}
char name[20];
printf("Enter the name of the employee to modify: ");
scanf("%s", name);
Employee *currentEmployee = head;
int found = 0;
while (currentEmployee != NULL) {
if (strcmp(currentEmployee->name, name) == 0) { // 找到要修改的节点
found = 1;
break;
}
currentEmployee = currentEmployee->next;
}
if (!found) { // 没有找到要修改的节点
printf("Employee not found.\n");
} else {
printf("Enter new age: ");
scanf("%d", ¤tEmployee->age);
printf("Enter new gender: ");
scanf("%s", currentEmployee->gender);
printf("Employee modified successfully.\n");
}
}
void searchEmployee() {
if (head == NULL) { // 链表为空
printf("No employees to search.\n");
return;
}
char name[20];
printf("Enter the name of the employee to search: ");
scanf("%s", name);
Employee *currentEmployee = head;
int found = 0;
while (currentEmployee != NULL) {
if (strcmp(currentEmployee->name, name) == 0) { // 找到要查找的节点
found = 1;
break;
}
currentEmployee = currentEmployee->next;
}
if (!found) { // 没有找到要查找的节点
printf("Employee not found.\n");
} else {
printf("Name: %s\n", currentEmployee->name);
printf("Age: %d\n", currentEmployee->age);
printf("Gender: %s\n", currentEmployee->gender);
}
}
void printAllEmployees() {
if (head == NULL) { // 链表为空
printf("No employees to print.\n");
return;
}
Employee *currentEmployee = head;
while (currentEmployee != NULL) {
printf("Name: %s\n", currentEmployee->name);
printf("Age: %d\n", currentEmployee->age);
printf("Gender: %s\n", currentEmployee->gender);
printf("\n");
currentEmployee = currentEmployee->next;
}
}
```
在该程序中,我们定义了一个员工结构体,包含员工的姓名、年龄和性别,并且还有一个指向下一个节点的指针。我们使用全局变量 `head` 来指向链表的头结点。
程序提供了以下操作:
- 添加员工信息
- 删除员工信息
- 修改员工信息
- 查找员工信息
- 打印所有员工信息
在添加员工信息时,我们先创建一个新的节点,然后从用户获取员工信息,并将新节点插入到链表头部。在删除员工信息时,我们先从用户获取要删除的员工姓名,然后遍历链表,找到对应的节点并删除。在修改员工信息时,我们要先从用户获取要修改的员工姓名,然后遍历链表,找到对应的节点并修改。在查找员工信息时,我们要先从用户获取要查找的员工姓名,然后遍历链表,找到对应的节点并输出。最后,在打印所有员工信息时,我们只需遍历链表并输出每个节点的信息即可。
我们可以根据需要对该程序进行修改,例如增加员工编号等信息。
阅读全文
相关推荐

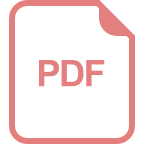
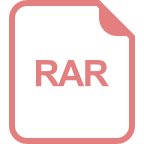
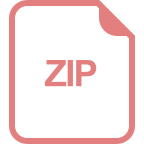
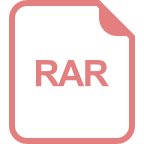
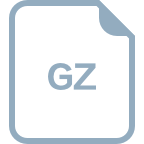
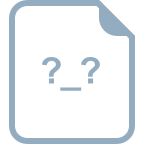
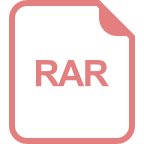
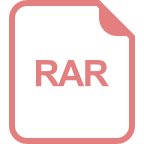
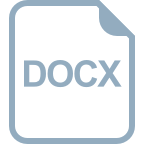
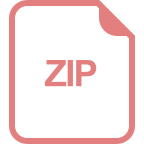
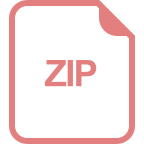
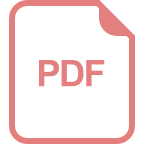
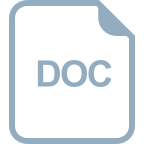