javascript编写一个脚本,当您单击“开始”按钮时运行。如果倒计时已经开始,“开始”按钮不应响应按下; 按下“暂停”按钮,倒计时应停止; 通过按“停止”按钮,倒计时应停止,字段值应重置为0。倒计时分钟和秒应每秒显示在页面上的相应输入字段中(带有标识符和min,sec); 当时间变为 0 分 0 秒时,倒计时应停止;并且时间倒计时到 0 分 0 秒时,显示用户设置的提醒文本,并将页面的背景色更改为用户指定的颜色。 有必要实现重新启动倒计时的功能(在这种情况下,您需要重置页面的背景颜色);
时间: 2024-02-18 13:04:27 浏览: 32
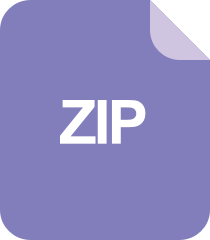
HTML弹出窗口上的自动单击按钮
好的,以下是实现您描述的功能的Javascript代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>倒计时</title>
<script type="text/javascript">
var countDown; // 定时器变量
var timeLeft = 0; // 剩余时间
var bgColor = ""; // 背景色
var alertMsg = ""; // 提醒文本
// 开始倒计时
function startCountDown() {
if (countDown) {
return; // 如果已经启动倒计时,则不响应按下
}
// 读取用户输入的时间和背景色、提醒文本
var minutes = parseInt(document.getElementById("minutes").value);
var seconds = parseInt(document.getElementById("seconds").value);
bgColor = document.getElementById("bgColor").value;
alertMsg = document.getElementById("alertMsg").value;
if (isNaN(minutes) || isNaN(seconds)) {
alert("请输入正确的时间");
return;
}
timeLeft = minutes * 60 + seconds; // 计算剩余时间
countDown = setInterval(updateTimeLeft, 1000); // 定时器每秒更新剩余时间
}
// 更新剩余时间
function updateTimeLeft() {
var minutes = Math.floor(timeLeft / 60);
var seconds = timeLeft % 60;
document.getElementById("minutes").value = minutes + "min";
document.getElementById("seconds").value = seconds + "sec";
timeLeft--;
if (timeLeft < 0) {
stopCountDown();
alert(alertMsg);
document.body.style.backgroundColor = bgColor; // 更改背景色
}
}
// 暂停倒计时
function pauseCountDown() {
clearInterval(countDown);
countDown = null;
}
// 停止倒计时
function stopCountDown() {
clearInterval(countDown);
countDown = null;
timeLeft = 0;
document.getElementById("minutes").value = "0min";
document.getElementById("seconds").value = "0sec";
document.body.style.backgroundColor = ""; // 重置背景色
}
</script>
</head>
<body>
<label for="minutes">分钟数:</label>
<input type="text" id="minutes" value="0min"><br>
<label for="seconds">秒数:</label>
<input type="text" id="seconds" value="0sec"><br>
<label for="bgColor">背景颜色:</label>
<input type="text" id="bgColor"><br>
<label for="alertMsg">提醒文本:</label>
<input type="text" id="alertMsg"><br>
<button onclick="startCountDown()">开始</button>
<button onclick="pauseCountDown()">暂停</button>
<button onclick="stopCountDown()">停止</button>
</body>
</html>
```
在上面的代码中,我们使用了一个定时器变量 `countDown` 来控制倒计时的启动、暂停和停止,使用 `setInterval()` 来每秒更新剩余时间。
在 `startCountDown()` 函数中,我们首先读取用户输入的时间、背景色和提醒文本,并计算出剩余时间。如果用户没有输入正确的时间,则弹出提示。
在 `updateTimeLeft()` 函数中,我们每秒更新剩余时间,并判断是否已经计时结束。如果计时结束,则停止倒计时、弹出提醒文本,并将页面的背景色更改为用户指定的颜色。
在 `pauseCountDown()` 函数中,我们停止定时器,暂停倒计时。
在 `stopCountDown()` 函数中,我们停止定时器、重置剩余时间和页面的背景色,停止倒计时。
阅读全文
相关推荐
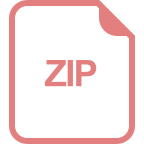
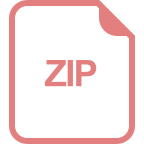


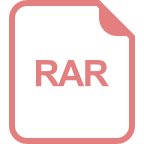
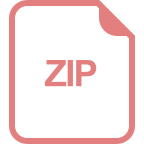
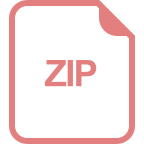
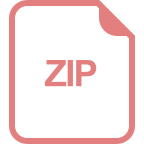
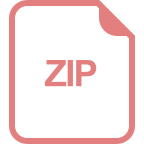
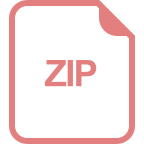
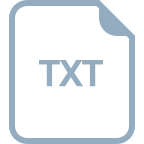
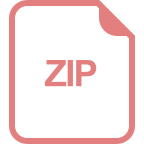
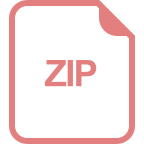
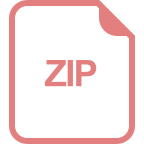
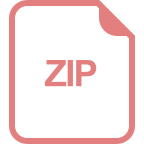
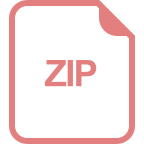
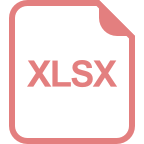