使用 python 编程实现固定步长的梯度下降法
时间: 2023-10-24 18:11:49 浏览: 38
以下是使用 Python 编程实现固定步长的梯度下降法的示例代码:
``` python
import numpy as np
def gradient_descent(X, y, theta, alpha, num_iters):
m = y.size
J_history = np.zeros(num_iters)
for i in range(num_iters):
h = X.dot(theta)
error = h - y
theta = theta - (alpha * (1/m) * X.T.dot(error))
J_history[i] = compute_cost(X, y, theta)
return (theta, J_history)
def compute_cost(X, y, theta):
m = y.size
h = X.dot(theta)
J = 1/(2*m) * np.sum(np.square(h-y))
return J
# 测试样例
X = np.array([[1, 2], [1, 3], [1, 4], [1, 5]])
y = np.array([7, 9, 11, 13])
theta = np.array([0, 0])
alpha = 0.01
num_iters = 1000
theta, J_history = gradient_descent(X, y, theta, alpha, num_iters)
print('theta:', theta)
print('J_history:', J_history)
```
在这个示例中,我们首先定义了一个 `gradient_descent` 函数来执行梯度下降算法,该函数接受训练数据、初始参数、学习率和迭代次数作为参数,并返回最终参数和每次迭代的代价函数值。然后我们定义了一个 `compute_cost` 函数来计算代价函数的值。
在测试样例中,我们使用了一个简单的数据集来测试我们的代码,数据集包含两个特征和一个目标变量。我们运行梯度下降算法来找到最适合数据集的线性回归模型,并输出最终参数和代价函数值。
相关推荐
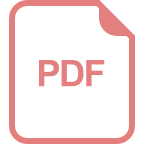
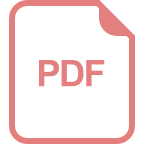
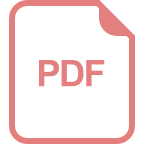














