使用opencv版C++,如何快速对一个容器内的点位进行去重
时间: 2023-12-26 15:05:58 浏览: 70
可以使用std::unique函数对容器内的点位进行去重,具体步骤如下:
1. 首先对容器内的点位进行排序,使用std::sort函数。
2. 然后使用std::unique函数对容器进行去重,可以使用容器的erase函数删除重复元素。
示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
struct Point {
int x, y;
bool operator==(const Point& other) const {
return x == other.x && y == other.y;
}
};
int main() {
std::vector<Point> points{{1, 2}, {3, 4}, {1, 2}, {5, 6}};
// 对容器内的点位进行排序
std::sort(points.begin(), points.end(), [](const Point& p1, const Point& p2) {
if (p1.x != p2.x) return p1.x < p2.x;
return p1.y < p2.y;
});
// 使用std::unique函数对容器进行去重
auto last = std::unique(points.begin(), points.end());
points.erase(last, points.end());
// 打印去重后的点位
for (const auto& p : points) {
std::cout << "(" << p.x << ", " << p.y << ")" << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
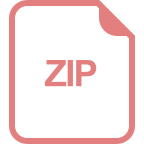
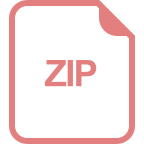
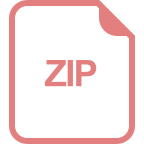
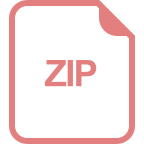
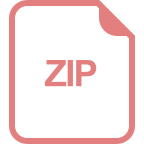
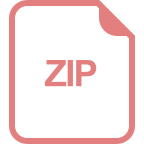
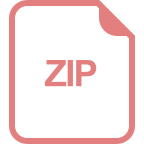
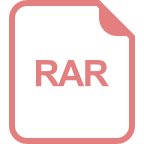
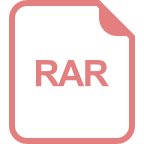
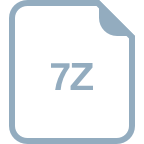
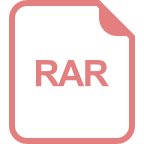
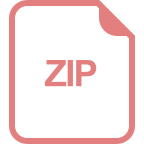
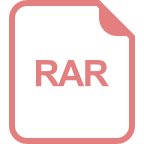
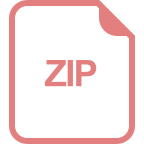