1,详细回答Java中关键字super和this的作用,并举例说明。 2.指出以下Java程序的错误,并改正。 【 class Test{ private int id; public void Test(){ Test(45); } public void Test(int id){ Test.id=id; } }】 3,简述以下程序出错的原因,并改正过来 【 public class t1 { public static void main(String[] args) { Number number=new Number(); } } abstract class Number{ public abstract int intValue(); } class Integer extends Number{ private int value; public int getValue() { return value; } public void setValue(int value) { this.value=value; } } 】
时间: 2024-01-26 08:04:51 浏览: 29
1. 关键字super和this在Java中的作用如下:
- super关键字用于调用父类的构造函数或成员变量、方法,可以使用super()调用父类构造函数,或使用super.field访问父类的成员变量,或使用super.method()调用父类的方法。
例如:
```
class Animal {
String name;
public Animal(String name) {
this.name = name;
}
}
class Cat extends Animal {
public Cat(String name) {
super(name);
}
}
```
- this关键字用于引用当前对象,可以使用this.field访问当前对象的成员变量,或使用this.method()调用当前对象的方法。
例如:
```
class Person {
String name;
int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void printInfo() {
System.out.println("name: " + this.name + ", age: " + this.age);
}
}
```
2. 以下是改正后的Java程序:
```
class Test{
private int id;
public Test(){
this(45);
}
public Test(int id){
this.id=id;
}
}
```
- 修改了Test()构造函数的定义,使用this(45)调用了另一个构造函数Test(int id)。
- 修改了Test(int id)构造函数的定义,使用this.id=id设置了私有变量id的值。
3. 以下是改正后的Java程序:
```
public class t1 {
public static void main(String[] args) {
Integer number = new Integer();
}
}
abstract class Number{
public abstract int intValue();
}
class Integer extends Number{
private int value;
public int intValue() {
return value;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value=value;
}
}
```
- 修改了类名Integer为MyInteger,因为Integer是Java中的一个类名。
- 实现了抽象类Number中的抽象方法intValue()。
- MyInteger类继承自抽象类Number,并实现了intValue()方法。
- 添加了MyInteger类中的getValue()和setValue()方法,用于获取和设置私有变量value的值。
相关推荐
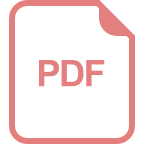
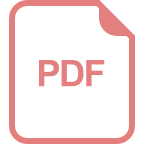














