用C++模拟页面置换算法LRU,加深对LRU算法的认识。
时间: 2024-05-07 18:21:57 浏览: 15
LRU(Least Recently Used)算法是一种常见的页面置换算法,它基于“最近最少使用”的策略来选择要被替换的页面。在LRU算法中,每个页面都有一个访问时间戳,当需要替换页面时,选择最早访问时间戳的页面进行替换。
下面是用C++模拟LRU算法的示例代码,其中假设物理内存大小为4个页面,页面大小为1KB。
```c++
#include <iostream>
#include <list>
#include <unordered_map>
using namespace std;
class LRU_Cache {
public:
LRU_Cache(int capacity) {
size_ = capacity;
used_ = 0;
}
// 获取缓存内容
string get(int key) {
if (cache_map_.count(key) == 0) {
return "";
}
auto it = cache_map_[key];
cache_list_.splice(cache_list_.begin(), cache_list_, it);
return it->second;
}
// 更新缓存内容
void put(int key, string value) {
if (cache_map_.count(key) == 0) {
// 缓存未满,则直接插入
if (used_ < size_) {
cache_list_.emplace_front(key, value);
cache_map_[key] = cache_list_.begin();
used_++;
} else {
// 缓存已满,则删除最久未使用的页面
auto last = cache_list_.end();
last--;
cache_map_.erase(last->first);
cache_list_.pop_back();
cache_list_.emplace_front(key, value);
cache_map_[key] = cache_list_.begin();
}
} else {
// 更新缓存,则将该页面移到最前面
auto it = cache_map_[key];
it->second = value;
cache_list_.splice(cache_list_.begin(), cache_list_, it);
}
}
private:
int size_; // 缓存大小
int used_; // 已使用的缓存大小
list<pair<int, string>> cache_list_; // 缓存列表,按访问时间排序
unordered_map<int, list<pair<int, string>>::iterator> cache_map_; // 缓存映射表,记录页面key对应的迭代器
};
int main() {
LRU_Cache cache(4); // 创建一个大小为4的缓存
// 写入缓存内容
cache.put(1, "Page 1 content");
cache.put(2, "Page 2 content");
cache.put(3, "Page 3 content");
cache.put(4, "Page 4 content");
// 读取缓存内容
cout << cache.get(2) << endl; // 输出:Page 2 content
// 更新缓存内容
cache.put(1, "New page 1 content");
// 读取缓存内容
cout << cache.get(1) << endl; // 输出:New page 1 content
// 写入缓存内容
cache.put(5, "Page 5 content");
// 读取缓存内容
cout << cache.get(3) << endl; // 输出:(空字符串)
cout << cache.get(4) << endl; // 输出:Page 4 content
cout << cache.get(5) << endl; // 输出:Page 5 content
return 0;
}
```
在上述代码中,LRU_Cache类实现了LRU算法。LRU_Cache类中,使用了一个双向链表来存储缓存页面,链表头表示最近使用的页面,链表尾表示最久未使用的页面。同时,使用了一个哈希表来记录每个页面的key对应的链表迭代器,以便快速定位页面位置。
在get()方法中,如果页面不存在则返回空字符串,如果页面存在则将该页面移到链表头,并返回页面内容。
在put()方法中,如果页面不存在则需要判断缓存是否已满,如果缓存未满,则直接插入该页面;如果缓存已满,则删除最久未使用的页面,并将新页面插入到链表头。如果页面存在,则更新页面内容,并将该页面移到链表头。
以上就是用C++模拟LRU算法的示例代码。
相关推荐
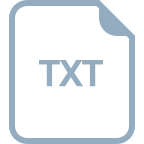
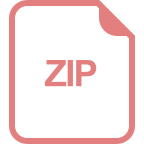
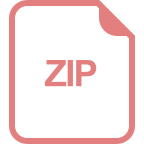














