Linux中 1)编写一个C程序,通过系统调用fork()创建一个子进程,利用无名管道的方式,将自己的学号从子进程向父进程传递;
时间: 2024-01-22 21:20:23 浏览: 27
可以参考以下代码实现:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#define BUF_SIZE 20
int main() {
char student_id[BUF_SIZE];
int fd[2];
pid_t pid;
if (pipe(fd) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
pid = fork();
if (pid < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid == 0) { // child process
close(fd[0]); // close read end of pipe
strcpy(student_id, "1234567890"); // replace with your student ID
write(fd[1], student_id, BUF_SIZE);
close(fd[1]); // close write end of pipe
exit(EXIT_SUCCESS);
} else { // parent process
close(fd[1]); // close write end of pipe
read(fd[0], student_id, BUF_SIZE);
printf("Student ID received from child process: %s\n", student_id);
close(fd[0]); // close read end of pipe
exit(EXIT_SUCCESS);
}
}
```
上述代码中,我们首先通过 `pipe()` 系统调用创建了一个无名管道。接着,我们使用 `fork()` 系统调用创建了一个子进程。在子进程中,我们关闭了管道的读端,然后将自己的学号写入管道的写端。在父进程中,我们关闭了管道的写端,然后从管道的读端中读取子进程发送的学号。最后,我们分别在父进程和子进程中关闭了管道的另一端,以确保资源得到释放。
需要注意的是,由于管道是半双工的,我们需要在父进程和子进程中分别关闭管道的一端。否则,会导致阻塞或者死锁等问题。
相关推荐
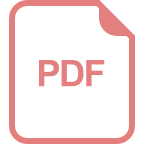
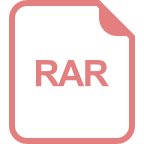
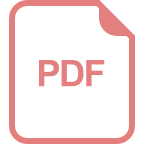














